JavaScript - Check two integers have opposite signs or not
JavaScript Bit Manipulation: Exercise-1 with Solution
Opposite Signs
Write a JavaScript program to check if two given integers have opposite signs.
Test Data:
(100, -100) -> "Signs are opposite"
(100, 100) -> "Signs are not opposite"
(‘100, 100) -> "Parameters value must be number!"
Sample Solution:
JavaScript Code:
// Define a function to check if the signs of two numbers are opposite
const opposite_Signs = (x, y) => {
// Check if the parameters are numbers
if ((typeof x!= "number") || (typeof y!= "number"))
{
return 'Parameters value must be number!' // Return an error message if parameters are not numbers
}
// Check if the signs of the two numbers are opposite
if ((x ^ y) < 0)
return true; // Return true if signs are opposite
else
return false; // Return false if signs are not opposite
}
x = 100 // Assign a value to variable x
y = -100 // Assign a value to variable y
result = opposite_Signs(x, y) // Call the function to check if signs are opposite
if (result === true)
console.log("Signs are opposite"); // Print a message if signs are opposite
else if (result === false)
console.log("Signs are not opposite"); // Print a message if signs are not opposite
else console.log(result); // Print the result if it's neither true nor false
x = 100 // Assign a value to variable x
y = 100 // Assign a value to variable y
result = opposite_Signs(x, y) // Call the function to check if signs are opposite
if (result === true)
console.log("Signs are opposite"); // Print a message if signs are opposite
else if (result === false)
console.log("Signs are not opposite"); // Print a message if signs are not opposite
else console.log(result); // Print the result if it's neither true nor false
x = '100' // Assign a value to variable x
y = 100 // Assign a value to variable y
result = opposite_Signs(x, y) // Call the function to check if signs are opposite
if (result === true)
console.log("Signs are opposite"); // Print a message if signs are opposite
else if (result === false)
console.log("Signs are not opposite"); // Print a message if signs are not opposite
else console.log(result); // Print the result if it's neither true nor false
Output:
Signs are opposite Signs are not opposite Parameters value must be number!
Flowchart:
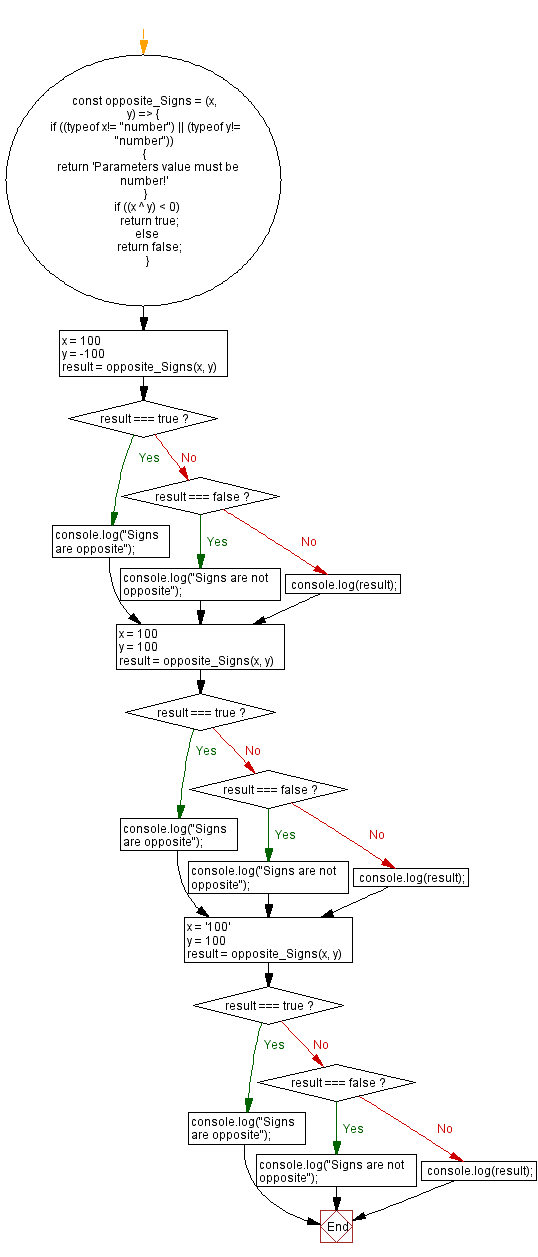
Live Demo:
See the Pen javascript-date-exercise-54 by w3resource (@w3resource) on CodePen.
* To run the code mouse over on Result panel and click on 'RERUN' button.*
Improve this sample solution and post your code through Disqus.
Previous: JavaScript Bit Manipulation Exercises Home.
Next: Swap two variables using bit manipulation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics