Java Testing Private methods with reflection: ExampleClass demonstration
8. Test Private Methods
Write a Java program to explore strategies for testing private methods in a class.
Sample Solution:
Java Code:
// ExampleClass.java
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
public class ExampleClass {
private int addTwoNumbers(int a, int b) {
return a + b;
}
// Public method that calls the private method
public int performAddition(int a, int b) {
return addTwoNumbers(a, b);
}
// Testing private method using reflection
public static int testPrivateMethod(ExampleClass instance, int a, int b)
throws NoSuchMethodException, InvocationTargetException, IllegalAccessException {
// Obtain the Class object for the class containing the private method
Class> clazz = instance.getClass();
// Specify the name and parameter types of the private method
Class[] parameterTypes = {int.class, int.class};
Method privateMethod = clazz.getDeclaredMethod("addTwoNumbers", parameterTypes);
// Make the private method accessible for testing
privateMethod.setAccessible(true);
// Invoke the private method on the provided instance
return (int) privateMethod.invoke(instance, a, b);
}
public static void main(String[] args) {
ExampleClass example = new ExampleClass();
// Test the public method that calls the private method
int result = example.performAddition(3, 5);
System.out.println("Result from public method: " + result);
try {
// Test the private method using reflection
int privateMethodResult = testPrivateMethod(example, 3, 5);
System.out.println("Result from private method: " + privateMethodResult);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
}
}
Sample Output:
Result from public method: 8 Result from private method: 8
Explanation:
In the exercise above,
- ExampleClass: Defines a class with a private method (addTwoNumbers) and a public method (performAddition) that calls the private method.
- testPrivateMethod Method: Uses reflection to test the private method. This method takes an instance of "ExampleClass", the parameters for the private method, and uses reflection to invoke the private method.
- main Method: Demonstrates how to test the public and private methods. The public method is tested directly, and the private method is tested using the testPrivateMethod utility.
Flowchart:
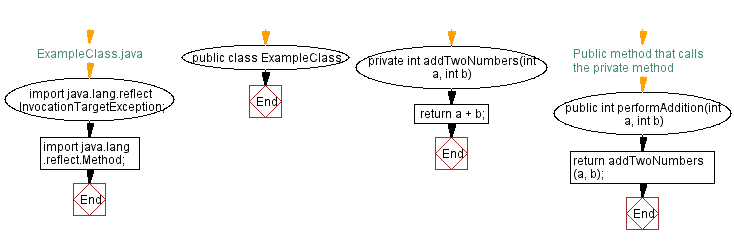
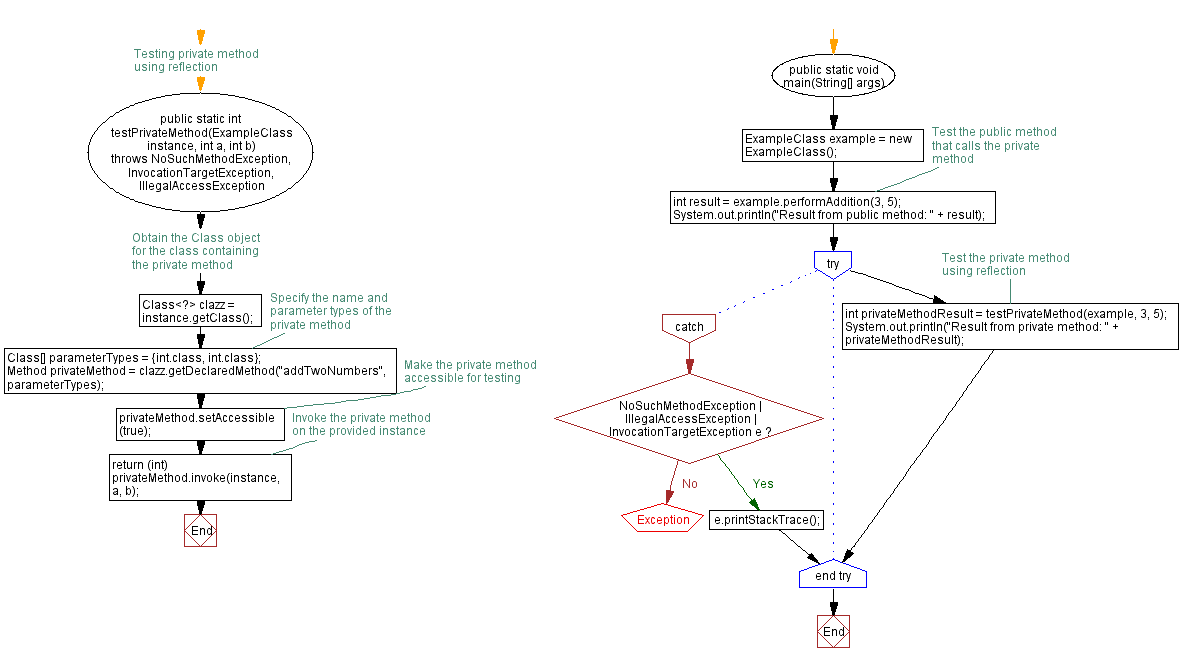
For more Practice: Solve these Related Problems:
- Write a Java program to create a test case that uses reflection to invoke and test private methods in a class.
- Write a Java program to design a test case that indirectly tests private methods through their public interfaces.
- Write a Java program to implement a test case that temporarily changes method visibility to facilitate private method testing.
- Write a Java program to construct a test case that employs reflection to access private fields and methods for thorough unit testing.
Go to:
PREV : Assert with Custom Error Message.
NEXT : Test Singleton in Multi-threaded Context.
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.