Java Application Integration testing with JUnit: UserService and OrderService Interaction
Write a Java program that create tests that verify the interaction between different components or modules in your application.
Sample Solution:
Java Code:
// ApplicationIntegrationTest.java
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
import static org.junit.Assert.assertEquals;
// Component 1: UserService
class UserService {
public String getUserFullName(int userId) {
// Simulate fetching user data from a database
// In a real application, this would involve interacting with a database or another service
return "Carl Mays"; // Assume a static user for simplicity
}
}
// Component 2: OrderService
class OrderService {
private UserService userService;
public OrderService(UserService userService) {
this.userService = userService;
}
public String getOrderDetails(int orderId) {
// Simulate fetching order details from a database
// In a real application, this would involve interacting with a database or another service
int userId = 1; // Assume a static user for simplicity
String userName = userService.getUserFullName(userId);
return "Order #123 for User: " + userName;
}
}
// JUnit Test Class
public class ApplicationIntegrationTest {
private UserService userService;
private OrderService orderService;
@Before
public void setUp() {
userService = new UserService();
orderService = new OrderService(userService);
}
@Test
public void testGetOrderDetails() {
// Test the interaction between UserService and OrderService
String orderDetails = orderService.getOrderDetails(123);
// Verify the result
assertEquals("Order #123 for User: Carl Mays", orderDetails);
}
public static void main(String[] args) {
// Run the JUnit test
Result result = JUnitCore.runClasses(ApplicationIntegrationTest.class);
// Check if there are any failures
if (result.getFailureCount() > 0) {
System.out.println("Test failed:");
// Print details of failures
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed successfully.");
}
}
}
Sample Output:
All tests passed successfully
Explanation:
The above Java code shows an integration test using JUnit to verify the interaction between two components in an application. Here's a brief explanation:
- Components:
- UserService: Simulates fetching user data. In a real application, this would involve interacting with a database or another service.
- OrderService: Uses "UserService" and simulates fetching order details. It involves interacting with a database or another service.
- Test Class (ApplicationIntegrationTest):
- Uses JUnit annotations (@Before, @Test) for setup and testing.
- setUp(): Initializes instances of "UserService" and "OrderService" before each test.
- testGetOrderDetails(): Tests the interaction between "UserService" and OrderService. It fetches order details using "OrderService" and verifies the result using "assertEquals".
- Main Method:
- main(): Runs the JUnit test using JUnitCore.runClasses().
- Checks for test failures and prints details if any.
Flowchart:

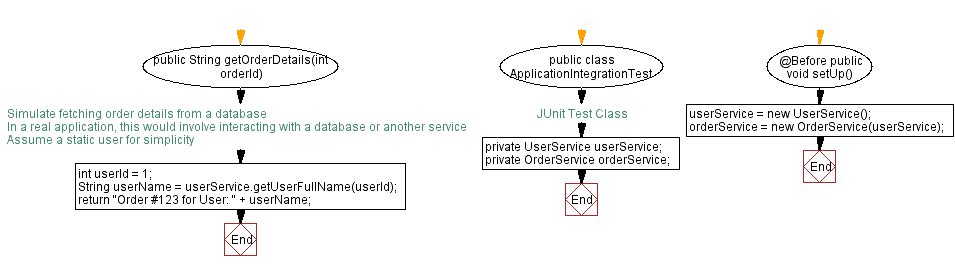
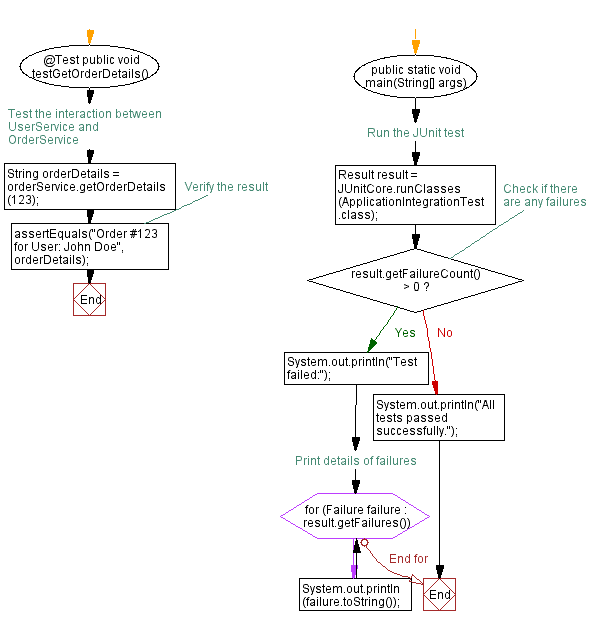
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Testing Java Singleton class for Multi-Threading with JUnit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics