Java Unit Test Case: Asserting Expected method returns
Write a Java unit test case to assert that a given method returns the expected value.
Sample Solution:
Java Code:
// Calculator.java
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
// CalculatorTest.java
import org.junit.Test;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
// Arrange
Calculator calculator = new Calculator();
// Act
int result = calculator.add(3, 5);
// Assert
assertEquals(8, result); // The expected value is 8
}
public static void main(String[] args) {
Result result = JUnitCore.runClasses(CalculatorTest.class);
// Check if there are any failures
if (result.getFailureCount() > 0) {
System.out.println("Test failed:");
// Print details of failures
for (Failure failure : result.getFailures()) {
System.out.println(failure.toString());
}
} else {
System.out.println("All tests passed successfully.");
}
}
}
Sample Output:
All tests passed successfully.
Explanation:
The above Java code is a JUnit test class (CalculatorTest) for testing a hypothetical "Calculator" class. The code uses JUnit's annotations and classes to define a test case and run the tests. Let break down the key components:
- Test Method:
- @Test Annotation: Indicates that the following method is a JUnit test case.
- Arrange: Sets up the test scenario, including creating an instance of the "Calculator" class.
- Act: Calls the "add()" method on the "Calculator" instance with specific input values (3 and 5).
- Assert: Uses the assertEquals method to verify that the result of the "add()" method is equal to the expected value (8).
- Main Method:
- JUnitCore.runClasses: Invokes JUnit to run all the test methods in the specified class (CalculatorTest.class).
- Result: Holds the result of the test execution, including information about passed and failed tests.
- Checking Failures: If there are failures, it prints a message indicating that the tests failed and provides details about each failure. Otherwise, it prints a success message.
Flowchart:
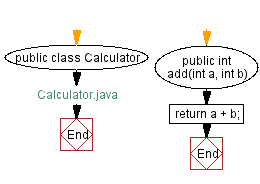
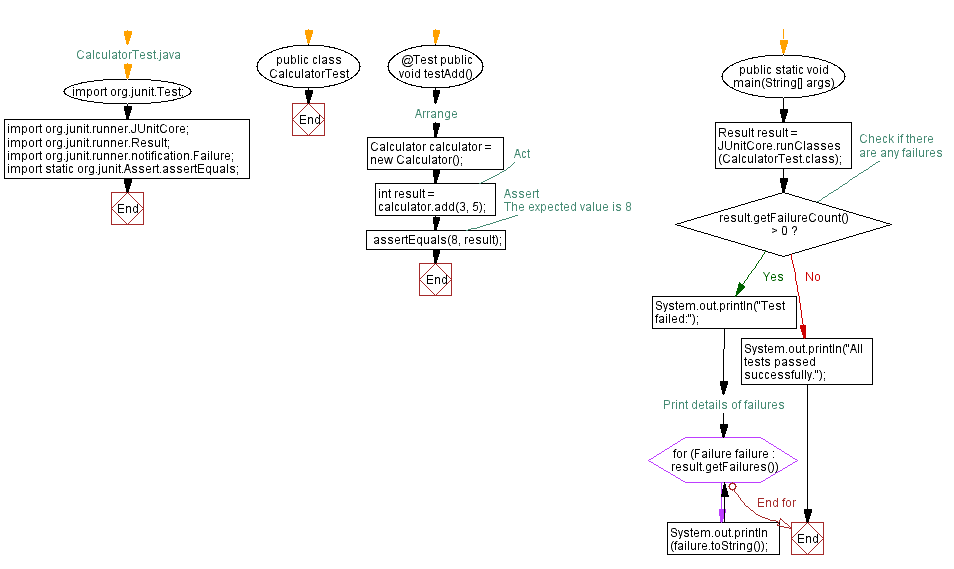
For more Practice: Solve these Related Problems:
- Write a Java program to create a unit test that asserts a method returns the expected value when provided with a typical input.
- Write a Java program to implement a JUnit test that compares the output of a method with a precomputed result using assertEquals.
- Write a Java program to design a test case that uses assertions to compare complex objects for deep equality.
- Write a Java program to construct a unit test that validates a function's return value with a custom delta for floating-point comparisons.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java Unit Test Exercises Home.
Next: Exception Testing.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.