Java: Read a string and returns after removing a specified character and its immediate left and right characters
Java String: Exercise-68 with Solution
Write Java program to read a string and return after removing specified characters and their immediate left and right adjacent characters.
Visual Presentation:
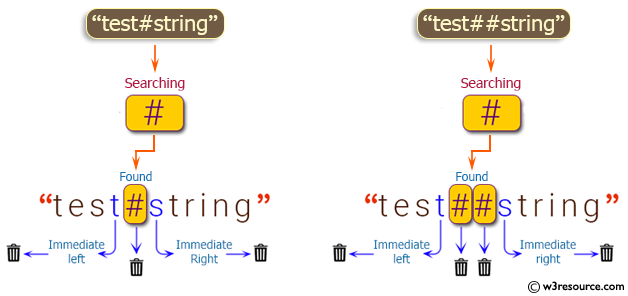
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to exclude '#' character considering some conditions from the input string
public String excludeHash(String stng) {
int len = stng.length(); // Get the length of the input string
String resultString = ""; // Initialize an empty string to store the result
// Iterate through each character in the input string
for (int i = 0; i < len; i++) {
// If the current character is the first character and it is not '#', append it to the resultString
if (i == 0 && stng.charAt(i) != '#')
resultString += stng.charAt(i);
// If the current character is not '#' and the previous character and current character both are not '#', append it to the resultString
if (i > 0 && stng.charAt(i) != '#' && stng.charAt(i - 1) != '#')
resultString += stng.charAt(i);
// If the current character is '#' and the previous character is not '#', remove the last character from resultString
if (i > 0 && stng.charAt(i) == '#' && stng.charAt(i - 1) != '#')
resultString = resultString.substring(0, resultString.length() - 1);
}
return resultString; // Return the modified string
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "test#string"; // Input string
// Display the given string and the new string using excludeHash method
System.out.println("The given strings is: " + str1);
System.out.println("The new string is: " + m.excludeHash(str1));
}
}
Sample Output:
The given strings is: test#string The new string is: testring The given strings is: test##string The new string is: testring The given strings is: test#the#string The new string is: teshtring
Flowchart:
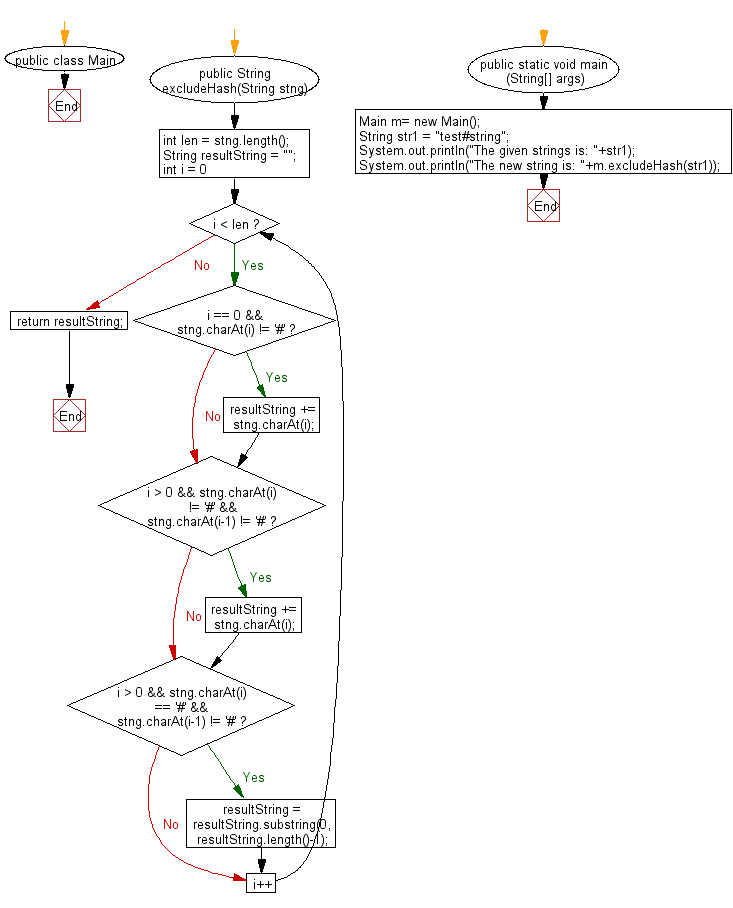
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to read a string and if one or both of the first two characters is equal to specified character return without those characters otherwise return the string unchanged.
Next: Write a Java program to return the substring that is between the first and last appearance of the substring 'toast' in the given string,or return the empty string if substirng 'toast' does not exists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics