Java: Read a string and if a substring of length two appears at both its beginning and end, return a string without the substring at the beginning otherwise, return the original string unchanged
Write a Java program to read a string. If a substring of length two appears at both its beginning and end, return a string without the substring at the beginning; otherwise, return the original string unchanged.
Visual Presentation:
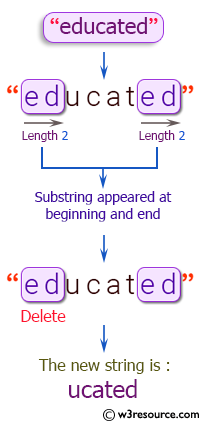
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to return a modified string based on certain conditions
public String withoutMacth(String str) {
int len = str.length(); // Get the length of the input string
if (len == 2) // If the length of the string is 2, return an empty string
return "";
if (len < 2) // If the length of the string is less than 2, return the original string
return str;
else {
if (str.substring(0, 2).equals(str.substring(len - 2, len)))
// If the substring of the first two characters is equal to the substring of the last two characters,
// return a modified string excluding the first two characters
return str.substring(2, len);
else
return str; // Otherwise, return the original string
}
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "educated"; // Input string
// Display the given string and the new string using withoutMacth method
System.out.println("The given strings is: " + str1);
System.out.println("The new string is: " + m.withoutMacth(str1));
}
}
Sample Output:
The given strings is: educated The new string is: ucated
Flowchart:
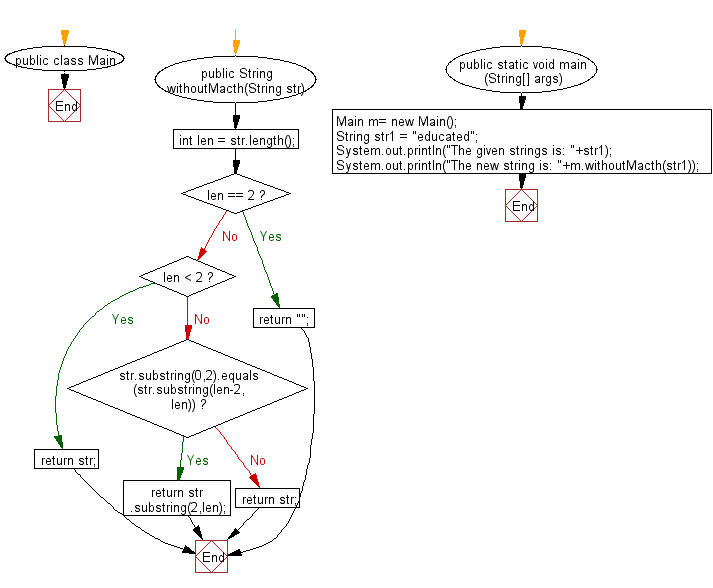
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to check whether the first two characters present at the end of a given string.
Next: Write a Java program to read a given string and if the first or last characters are same return the string without those characters otherwise return the string unchanged.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics