Java: New string taking specified number of characters from first and last position of a given string
Java String: Exercise-61 with Solution
Write a Java program to create a new string taking specified number of characters from first and last position of a given string.
Visual Presentation:
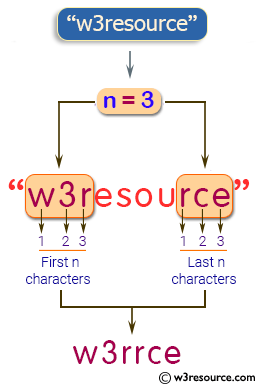
Sample Solution:
Java Code:
import java.util.*;
// Define a class named Main
public class Main {
// Method to return a string formed by taking 'n' characters from the beginning and 'n' characters from the end
public String nTwice(String str, int n) {
return str.substring(0, n) + str.substring(str.length() - n, str.length());
}
// Main method to execute the program
public static void main(String[] args) {
Main m = new Main(); // Create an instance of the Main class
String str1 = "Welcome"; // String
int n1 = 3; // Integer 'n'
// Display the given string, the given number, and the new string formed using nTwice method
System.out.println("The given strings is: " + str1);
System.out.println("The given numbers is: " + n1);
System.out.println("The new string is: " + m.nTwice(str1, n1));
}
}
Sample Output:
The given strings is: Welcome The given numbers is: 3 The new string is: Welome
Flowchart:
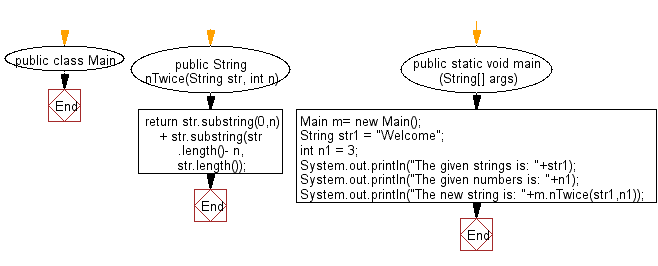
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to read two strings append them together and return the result. If the strings are different lengths, remove characters from the beginning of longer string and make them equal length.
Next: Write a Java program to read a string and return true if "good" appears starting at index 0 or 1 in the given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/string/java-string-exercise-61.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics