Java: Remove all adjacent duplicates recursively from a given string
Java String: Exercise-55 with Solution
Write a Java program to remove all adjacent duplicates recursively from a given string.
Visual Presentation:
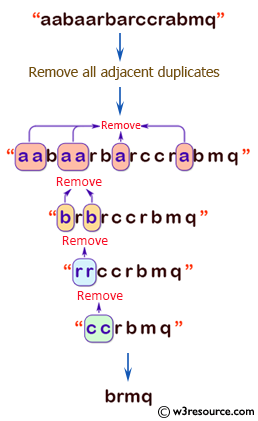
Sample Solution:
Java Code:
// Import necessary Java utilities and packages.
import java.util.*;
import java.lang.*;
import java.io.*;
// Define a class named Main.
class Main {
// Method to remove all adjacent duplicates from the string.
public static void check(String str) {
// Check if the length of the string is less than or equal to 1.
if (str.length() <= 1) {
// Print the string and return if the length is 1 or less.
System.out.println(str);
return;
}
// Initialize variables.
String n = new String();
int count = 0;
// Loop through the string to remove adjacent duplicates.
for (int i = 0; i < str.length(); i++) {
// Check for adjacent duplicates and skip them.
while (i < str.length() - 1 && str.charAt(i) == str.charAt(i + 1)) {
if (i < str.length() - 2 && str.charAt(i) != str.charAt(i + 2))
i += 2;
else
i++;
count++;
}
// Add non-duplicate characters to the new string.
if (i != str.length() - 1)
n = n + str.charAt(i);
else {
if (i == str.length() - 1 && str.charAt(i) != str.charAt(i - 1))
n = n + str.charAt(i);
}
}
// Recursively check for more adjacent duplicates.
if (count > 0)
check(n);
else
// Print the final string after removing adjacent duplicates.
System.out.println(n);
}
// Main method to execute the program.
public static void main(String[] args) {
// Define a sample string for testing.
String ab = "aabaarbarccrabmq";
System.out.println("The given string is: " + ab);
System.out.println("The new string after removing all adjacent duplicates is:");
// Call the check method to remove adjacent duplicates.
check(ab);
}
}
Sample Output:
The given string is: aabaarbarccrabmq The new string after removing all adjacent duplicates is: brmq
Flowchart: 1
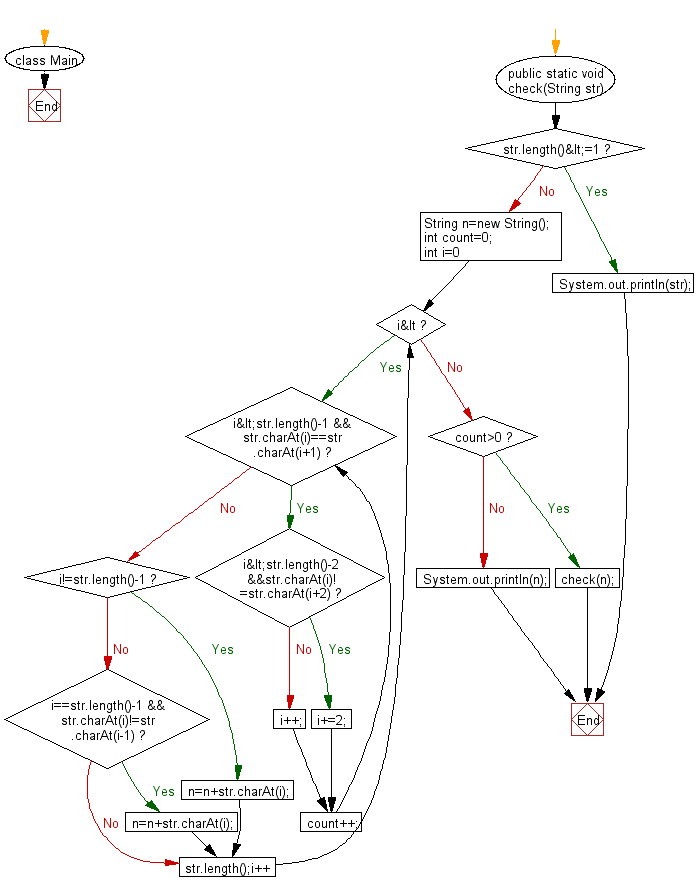
Flowchart: 2
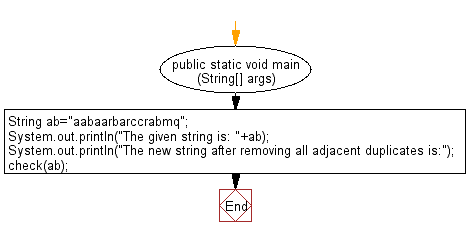
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to find the smallest window in a string containing all characters of another string.
Next: Write a Java program to append two given strings such that, if the concatenation creates a double characters then omit one of the characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/string/java-string-exercise-55.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics