Java: Reverse words in a given string
Java String: Exercise-45 with Solution
Write a Java program to reverse words in a given string.
Visual Presentation:
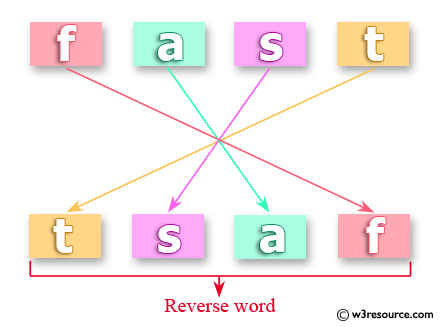
Sample Solution-1:
Java Code:
// Importing necessary Java utilities.
import java.util.*;
// Define a class named Main.
public class Main {
// Method to reverse words in a given string.
public static String WordsInReverse(String str1) {
// Create a StringBuilder object and reverse the entire string.
StringBuilder sb = new StringBuilder(str1);
String StrRev = sb.reverse().toString();
// Split the reversed string into words.
String[] words = StrRev.split(" ");
// Create a StringBuilder to store the reversed words.
StringBuilder reverse = new StringBuilder();
// Iterate through each word, reverse it, and append it to the result string.
for (String tmp: words) {
sb = new StringBuilder(tmp);
reverse.append(sb.reverse() + " ");
}
// Remove the trailing space and return the reversed words string.
reverse.deleteCharAt(reverse.length() - 1);
return reverse.toString();
}
// Main method to execute the program.
public static void main(String[] args) {
String str1 = "Reverse words in a given string"; // Given input string.
// Display the given string.
System.out.println("The given string is: " + str1);
// Display the string after reversing the words.
System.out.println("The new string after reversing the words: " + WordsInReverse(str1));
}
}
Sample Output:
The given string is: Reverse words in a given string The new string after reversed the words: string given a in words Reverse
Flowchart:
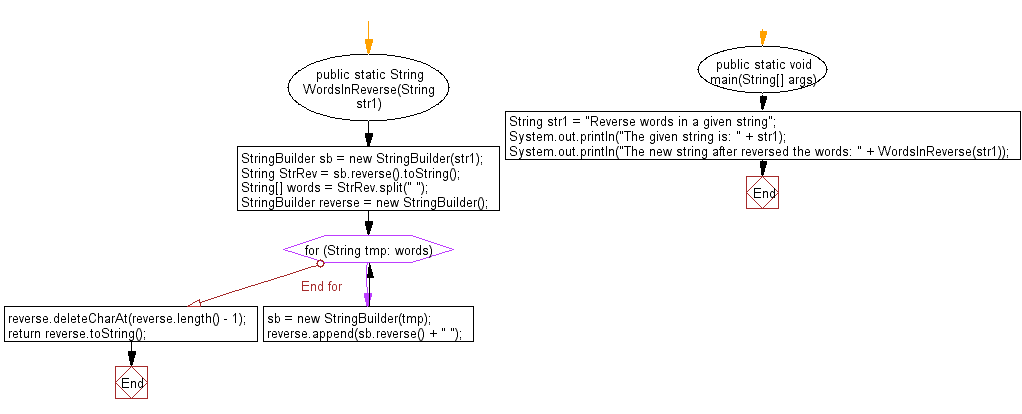
Sample Solution-2:
Main.java Code:
//MIT License: https://bit.ly/35gZLa3
import java.util.concurrent.TimeUnit;
public class Main {
private static final String TEXT = "My high school, the Illinois Mathematics and Science Academy, "
+ "showed me that anything is possible and that you're never too young to think big. "
+ "At 15, I worked as a computer programmer at the Fermi National Accelerator Laboratory, "
+ "or Fermilab. After graduating, I attended Stanford for a degree in economics and "
+ "computer science.";
public static void main(String[] args) {
System.out.println("Input text: \n" + TEXT + "\n");
System.out.println("Reverse words in String via StringBuilder:");
long startTimeV1 = System.nanoTime();
String reversedV1 = Strings.reverseWordsV1(TEXT);
displayExecutionTime(System.nanoTime() - startTimeV1);
System.out.println("Reversed: \n" + reversedV1);
System.out.println();
System.out.println("Reverse words in String using Java 8 functional-style:");
long startTimeV2 = System.nanoTime();
String reversedV2 = Strings.reverseWordsV2(TEXT);
displayExecutionTime(System.nanoTime() - startTimeV2);
System.out.println("Reversed: \n" + reversedV2);
System.out.println();
System.out.println("Reverse String via StringBuilder:");
long startTimeV3 = System.nanoTime();
String reversedV3 = Strings.reverse(TEXT);
displayExecutionTime(System.nanoTime() - startTimeV3);
System.out.println("Reversed: \n" + reversedV3);
}
private static void displayExecutionTime(long time) {
System.out.println("Execution time: " + time + " ns" + " ("
+ TimeUnit.MILLISECONDS.convert(time, TimeUnit.NANOSECONDS) + " ms)");
}
}
Strings.java Code:
//MIT License: https://bit.ly/35gZLa3
import java.util.regex.Pattern;
import java.util.stream.Collectors;
public final class Strings {
private static final Pattern PATTERN = Pattern.compile(" +");
private static final String WHITESPACE = " ";
private Strings() {
throw new AssertionError("Cannot be instantiated");
}
public static String reverseWordsV1(String str) {
if (str == null || str.isEmpty()) {
// or throw IllegalArgumentException
return "";
}
String[] words = str.split(WHITESPACE);
StringBuilder reversedString = new StringBuilder();
for (String word : words) {
StringBuilder reverseWord = new StringBuilder();
for (int i = word.length() - 1; i >= 0; i--) {
reverseWord.append(word.charAt(i));
}
reversedString.append(reverseWord).append(WHITESPACE);
}
return reversedString.toString();
}
public static String reverseWordsV2(String str) {
// or throw IllegalArgumentException
if (str == null || str.isEmpty()) {
return "";
}
return PATTERN.splitAsStream(str)
.map(w -> new StringBuilder(w).reverse())
.collect(Collectors.joining(WHITESPACE));
}
public static String reverse(String str) {
// or throw IllegalArgumentException
if (str == null || str.isEmpty()) {
return "";
}
return new StringBuilder(str).reverse().toString();
}
}
Sample Output:
Input text: My high school, the Illinois Mathematics and Science Academy, showed me that anything is possible and that you're never too young to think big. At 15, I worked as a computer programmer at the Fermi National Accelerator Laboratory, or Fermilab. After graduating, I attended Stanford for a degree in economics and computer science. Reverse words in String via StringBuilder: Execution time: 2670238 ns (2 ms) Reversed: yM hgih ,loohcs eht sionillI scitamehtaM dna ecneicS ,ymedacA dewohs em taht gnihtyna si elbissop dna taht er'uoy reven oot gnuoy ot kniht .gib tA ,51 I dekrow sa a retupmoc remmargorp ta eht imreF lanoitaN rotareleccA ,yrotarobaL ro .balimreF retfA ,gnitaudarg I dednetta drofnatS rof a eerged ni scimonoce dna retupmoc .ecneics Reverse words in String using Java 8 functional-style: Execution time: 143280080 ns (143 ms) Reversed: yM hgih ,loohcs eht sionillI scitamehtaM dna ecneicS ,ymedacA dewohs em taht gnihtyna si elbissop dna taht er'uoy reven oot gnuoy ot kniht .gib tA ,51 I dekrow sa a retupmoc remmargorp ta eht imreF lanoitaN rotareleccA ,yrotarobaL ro .balimreF retfA ,gnitaudarg I dednetta drofnatS rof a eerged ni scimonoce dna retupmoc .ecneics Reverse String via StringBuilder: Execution time: 91373 ns (0 ms) Reversed: .ecneics retupmoc dna scimonoce ni eerged a rof drofnatS dednetta I ,gnitaudarg retfA .balimreF ro ,yrotarobaL rotareleccA lanoitaN imreF eht ta remmargorp retupmoc a sa dekrow I ,51 tA .gib kniht ot gnuoy oot reven er'uoy taht dna elbissop si gnihtyna taht em dewohs ,ymedacA ecneicS dna scitamehtaM sionillI eht ,loohcs hgih yM
Flowchart:
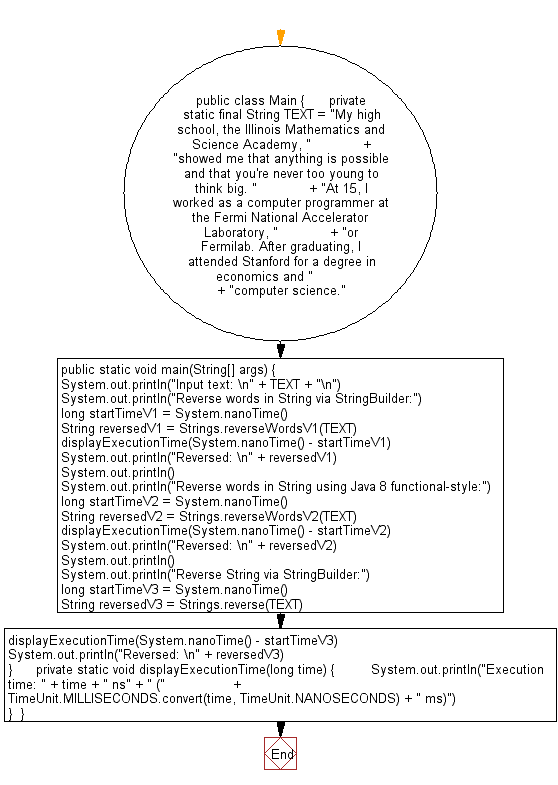
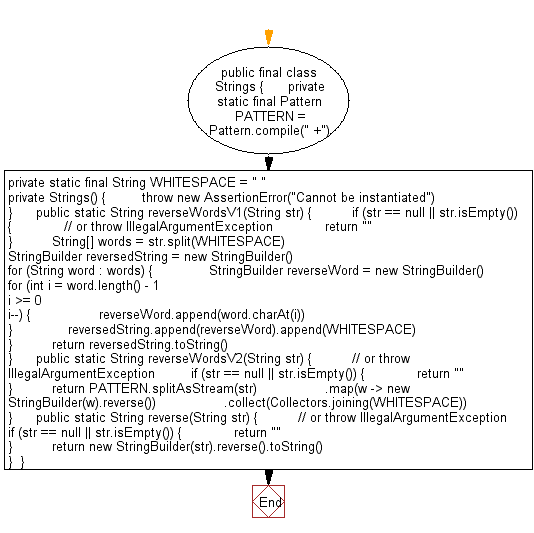
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to reverse a string using recursion.
Next: Write a Java program to reverse every word in a string using methods.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics