Java: Find the maximum occurring character in a string
Write a Java program to find the character in a string that occurs the most frequently.
Sample Solution:
Java Code:
// Importing necessary Java utilities.
import java.util.*;
// Define a class named Main.
public class Main {
// Define a constant for the size of the character set.
static final int N = 256;
// Method to find the character with the maximum occurrence in the string.
static char MaxOccuringChar(String str1) {
int ctr[] = new int[N]; // Array to count occurrences of each character.
int l = str1.length(); // Length of the given string.
// Loop through the string to count occurrences of each character.
for (int i = 0; i < l; i++)
ctr[str1.charAt(i)]++;
int max = -1; // Variable to store maximum occurrence.
char result = ' '; // Variable to store the character with the maximum occurrence.
// Loop through the string to find the character with the maximum occurrence.
for (int i = 0; i < l; i++) {
if (max < ctr[str1.charAt(i)]) {
max = ctr[str1.charAt(i)];
result = str1.charAt(i);
}
}
return result; // Return the character with the maximum occurrence.
}
// Main method to execute the program.
public static void main(String[] args) {
String str1 = "test string"; // Given input string.
// Display the given string.
System.out.println("The given string is: " + str1);
// Display the character with the maximum occurrence in the given string.
System.out.println("Max occurring character in the given string is: " + MaxOccuringChar(str1));
}
}
Sample Output:
The given string is: test string Max occurring character in the given string is: t
Flowchart:
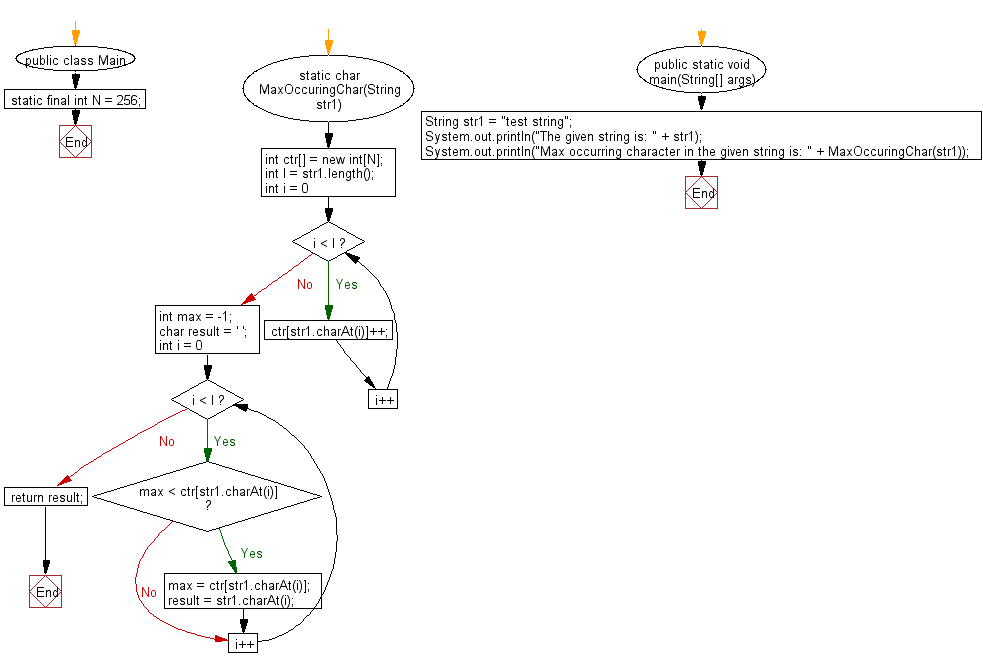
Find the character with the most appearances.
Main.java Code:
//MIT License: https://bit.ly/35gZLa3
import java.util.concurrent.TimeUnit;
public class Main {
private static final String TEXT = "My high school, the Illinois Mathematics and Science Academy, "
+ "showed me that anything is possible and that you're never too young to think big. "
+ "At 15, I worked as a computer programmer at the Fermi National Accelerator Laboratory, "
+ "or Fermilab. After graduating, I attended Stanford for a degree in economics and "
+ "computer science.";
public static void main(String[] args) {
System.out.println("Input text: \n" + TEXT + "\n");
System.out.println("HashMap based solution:");
long startTimeV1 = System.nanoTime();
Pair pairV1 = Strings.maxOccurenceCharacterV1(TEXT);
displayExecutionTime(System.nanoTime() - startTimeV1);
System.out.println("Character: " + pairV1.character);
System.out.println("Occurrences :" + pairV1.occurrences);
System.out.println();
System.out.println("ASCII codes based solution:");
long startTimeV2 = System.nanoTime();
Pair pairV2 = Strings.maxOccurenceCharacterV2(TEXT);
displayExecutionTime(System.nanoTime() - startTimeV2);
System.out.println("Character: " + pairV2.character);
System.out.println("Occurrences :" + pairV2.occurrences);
System.out.println();
System.out.println("Java 8, functional-style solution:");
long startTimeV3 = System.nanoTime();
Pair pairV3 = Strings.maxOccurenceCharacterV3(TEXT);
displayExecutionTime(System.nanoTime() - startTimeV3);
System.out.println("Character: " + pairV3.character);
System.out.println("Occurrences :" + pairV3.occurrences);
}
private static void displayExecutionTime(long time) {
System.out.println("Execution time: " + time + " ns" + " ("
+ TimeUnit.MILLISECONDS.convert(time, TimeUnit.NANOSECONDS) + " ms)");
}
}
Pair.java Code:
//MIT License: https://bit.ly/35gZLa3
public final class Pair<V, C> {
final V character;
final C occurrences;
public Pair(V character, C occurrences) {
this.character = character;
this.occurrences = occurrences;
}
static <V, C> Pair<V, C> of(V character, C occurrences) {
return new Pair<>(character, occurrences);
}
@Override
public int hashCode() {
return character.hashCode() ^ character.hashCode();
}
@Override
public boolean equals(Object o) {
if (!(o instanceof Pair)) {
return false;
}
Pair obj = (Pair) o;
return this.character.equals(obj.character)
&& this.occurrences.equals(obj.occurrences);
}
}
Strings.java Code:
//MIT License: https://bit.ly/35gZLa3
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import static java.util.Map.Entry.comparingByValue;
import static java.util.stream.Collectors.counting;
import static java.util.stream.Collectors.groupingBy;
public final class Strings {
private static final int EXTENDED_ASCII_CODES = 256;
private Strings() {
throw new AssertionError("Cannot be instantiated");
}
public static Pair<Character, Integer> maxOccurenceCharacterV1(String str) {
if (str == null || str.isEmpty()) {
// or throw IllegalArgumentException
return Pair.of(Character.MIN_VALUE, -1);
}
Map<Character, Integer> counter = new HashMap<>();
char[] chStr = str.toCharArray();
for (int i = 0; i < chStr.length; i++) {
char currentCh = chStr[i];
if (!Character.isWhitespace(currentCh)) { // ignore spaces
Integer noCh = counter.get(currentCh);
if (noCh == null) {
counter.put(currentCh, 1);
} else {
counter.put(currentCh, ++noCh);
}
}
}
int maxOccurrences = Collections.max(counter.values());
char maxCharacter = Character.MIN_VALUE;
for (Entry<Character, Integer> entry : counter.entrySet()) {
if (entry.getValue() == maxOccurrences) {
maxCharacter = entry.getKey();
}
}
return Pair.of(maxCharacter, maxOccurrences);
}
public static Pair<Character, Integer> maxOccurenceCharacterV2(String str) {
if (str == null || str.isEmpty()) {
// or throw IllegalArgumentException
return Pair.of(Character.MIN_VALUE, -1);
}
int maxOccurrences = -1;
char maxCharacter = Character.MIN_VALUE;
char[] chStr = str.toCharArray();
int[] asciiCodes = new int[EXTENDED_ASCII_CODES];
for (int i = 0; i < chStr.length; i++) {
char currentCh = chStr[i];
if (!Character.isWhitespace(currentCh)) { // ignoring space
int code = (int) currentCh;
asciiCodes[code]++;
if (asciiCodes[code] > maxOccurrences) {
maxOccurrences = asciiCodes[code];
maxCharacter = currentCh;
}
}
}
return Pair.of(maxCharacter, maxOccurrences);
}
public static Pair<Character, Long> maxOccurenceCharacterV3(String str) {
if (str == null || str.isEmpty()) {
// or throw IllegalArgumentException
return Pair.of(Character.MIN_VALUE, -1L);
}
return str.chars()
.filter(c -> Character.isWhitespace(c) == false) // ignoring space
.mapToObj(c -> (char) c)
.collect(groupingBy(c -> c, counting()))
.entrySet()
.stream()
.max(comparingByValue())
.map(p -> Pair.of(p.getKey(), p.getValue()))
.orElse(Pair.of(Character.MIN_VALUE, -1L));
}
}
Sample Output:
Input text: My high school, the Illinois Mathematics and Science Academy, showed me that anything is possible and that you're never too young to think big. At 15, I worked as a computer programmer at the Fermi National Accelerator Laboratory, or Fermilab. After graduating, I attended Stanford for a degree in economics and computer science. HashMap based solution: Execution time: 3438752 ns (3 ms) Character: e Occurrences :29 ASCII codes based solution: Execution time: 215566 ns (0 ms) Character: e Occurrences :29 Java 8, functional-style solution: Execution time: 120723045 ns (120 ms) Character: e Occurrences :29
Flowchart:
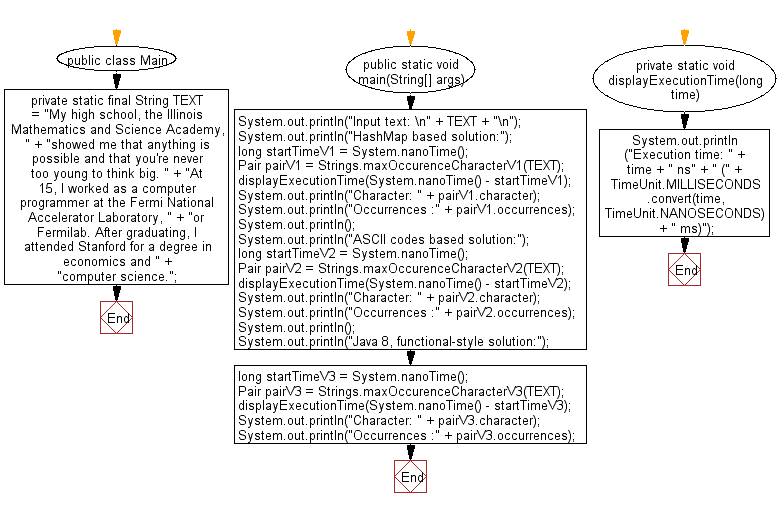
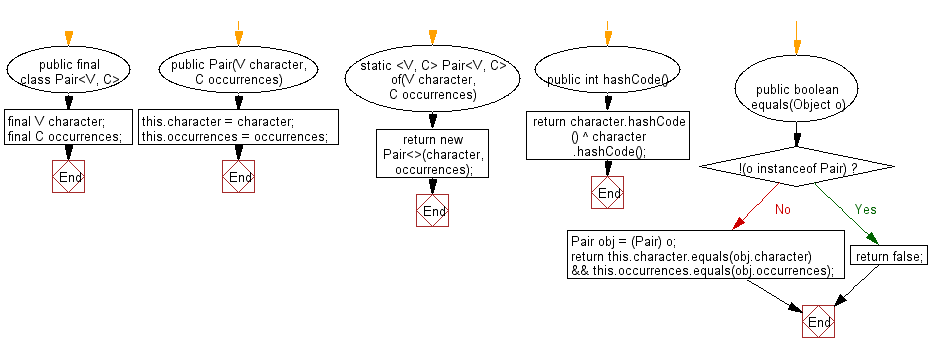
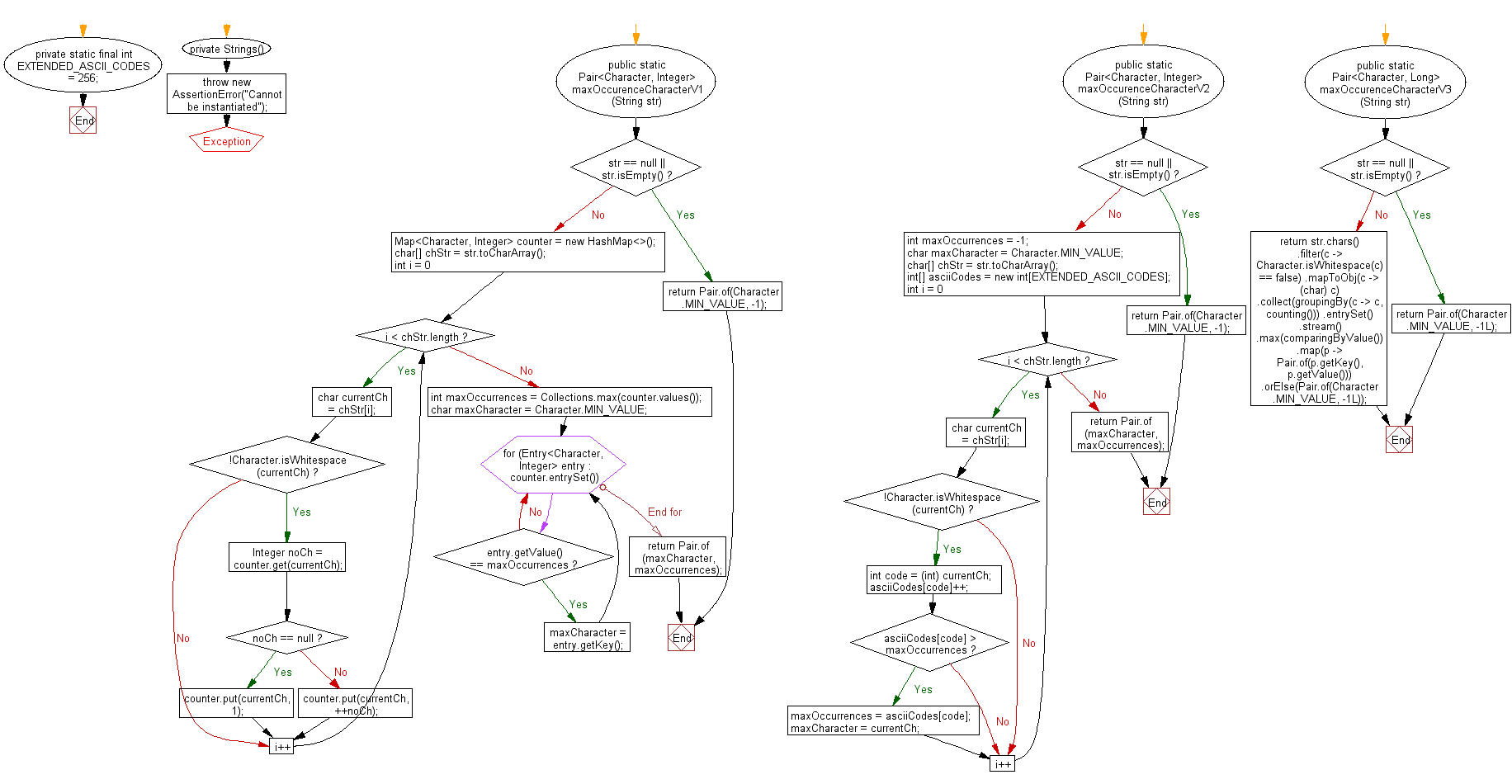
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to print list items containing all characters of a given word.
Next: Write a Java program to reverse a string using recursion
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics