Java: Find first non repeating character in a string
Java String: Exercise-39 with Solution
Write a Java program to find the first non-repeating character in a string.
Visual Presentation:
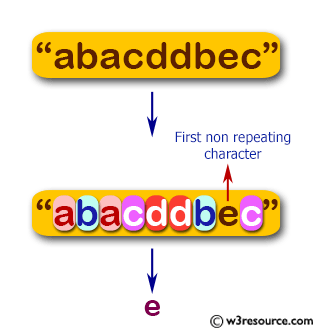
Sample Solution:
Java Code:
// Importing necessary Java utilities.
import java.util.*;
// Define a class named Main.
public class Main {
// Main method to execute the program.
public static void main(String[] args) {
// Declare and initialize a string variable.
String str1 = "gibblegabbler";
// Print the original string.
System.out.println("The given string is: " + str1);
// Loop through each character of the string.
for (int i = 0; i < str1.length(); i++) {
// Assume the character at index 'i' is unique initially.
boolean unique = true;
// Loop through the string again to compare characters.
for (int j = 0; j < str1.length(); j++) {
// Check if the characters at positions 'i' and 'j' are the same but not at the same index.
if (i != j && str1.charAt(i) == str1.charAt(j)) {
// If found, set unique to false and break the loop.
unique = false;
break;
}
}
// If the character at index 'i' is unique, print it and exit the loop.
if (unique) {
System.out.println("The first non-repeated character in the String is: " + str1.charAt(i));
break;
}
}
}
}
Sample Output:
The given string is: gibblegabbler The first non repeated character in String is: i
Flowchart:
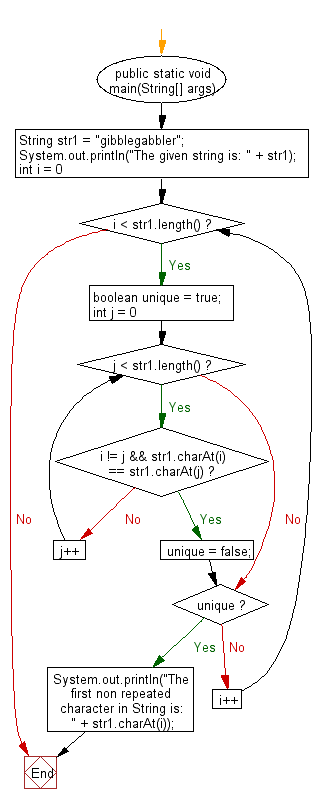
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to print after removing duplicates from a given string.
Next: Write a Java program to divide a string in n equal parts.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics