Java: Get a substring of a given string between two specified positions
Java String: Exercise-27 with Solution
Write a Java program to get a substring of a given string at two specified positions.
Visual Presentation:
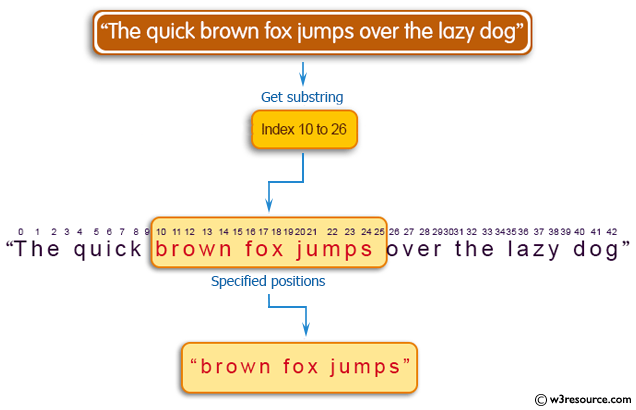
Sample Solution:
Java Code:
// Define a public class named Exercise27.
public class Exercise27 {
// Define the main method.
public static void main(String[] args) {
// Declare and initialize a string variable.
String str = "The quick brown fox jumps over the lazy dog.";
// Get a substring of the above string starting from
// index 10 and ending at index 26.
String new_str = str.substring(10, 26);
// Display the original and the extracted substring for comparison.
System.out.println("old = " + str);
System.out.println("new = " + new_str);
}
}
Sample Output:
old = The quick brown fox jumps over the lazy dog. new = brown fox jumps
Flowchart:
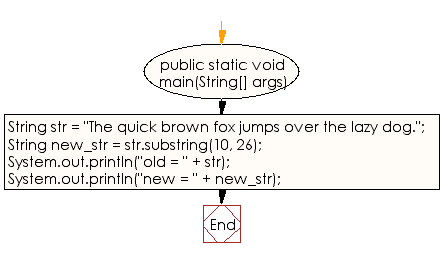
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to check whether a given string starts with the contents of another string.
Next: Write a Java program to create a character array containing the contents of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/string/java-string-exercise-27.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics