Java: Find whether a region in the current string matches a region in another string
Write a Java program to find out whether a region in the current string matches a region in another string.
Sample Solution:
Java Code:
// Define a public class named Exercise23.
public class Exercise23 {
// Define the main method.
public static void main(String[] args) {
// Define two string variables.
String str1 = "Shanghai Houston Colorado Alexandria";
String str2 = "Alexandria Colorado Houston Shanghai";
// Determine whether characters 0 through 7 in str1
// match characters 28 through 35 in str2.
boolean match1 = str1.regionMatches(0, str2, 28, 8);
// Determine whether characters 9 through 15 in str1
// match characters 9 through 15 in str2.
boolean match2 = str1.regionMatches(9, str2, 9, 8);
// Display the results of the regionMatches method calls.
System.out.println("str1[0 - 7] == str2[28 - 35]? " + match1);
System.out.println("str1[9 - 15] == str2[9 - 15]? " + match2);
}
}
Sample Output:
str1[0 - 7] == str2[28 - 35]? true str1[9 - 15] == str2[9 - 15]? false
Flowchart:
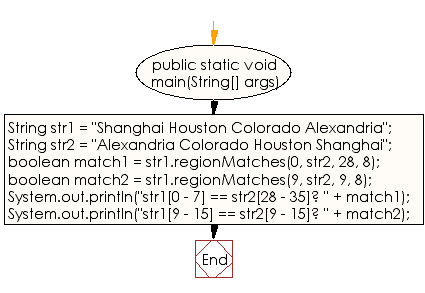
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a java program to get the length of a given string.
Next: Write a Java program to replace a specified character with another character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics