Java: Print current date and time in the specified format
Java String: Exercise-15 with Solution
Write a Java program to print the current date and time in the specified format.
Visual Presentation:
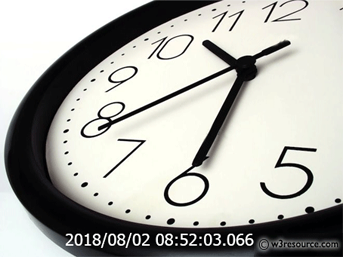
Sample Solution:
Java Code:
// Import the Calendar class from the java.util package.
import java.util.Calendar;
// Define a public class named Exercise15.
public class Exercise15 {
// Define the main method.
public static void main(String[] args) {
// Get an instance of the Calendar class representing the current date and time.
Calendar c = Calendar.getInstance();
// Print the message indicating current date and time.
System.out.println("Current Date and Time :");
// Print the formatted date (month day, year) using the specified format.
System.out.format("%tB %te, %tY%n", c, c, c);
// Print the formatted time (hour:minute am/pm) using the specified format.
System.out.format("%tl:%tM %tp%n", c, c, c);
}
}
Sample Output:
Current Date and Time : June 19, 2017 3:13 pm
N.B. : The current date and time will change according to your system date and time.
Flowchart:
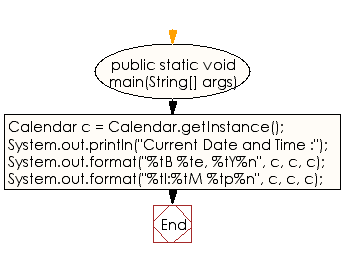
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to compare a given string to another string, ignoring case considerations.
Next: Write a Java program to get the contents of a given string as a byte array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/string/java-string-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics