Java: Count duplicate characters in a String
Write a Java program to count the number of characters (alphanumeric only) that occur more than twice in a given string.
Visual Presentation:
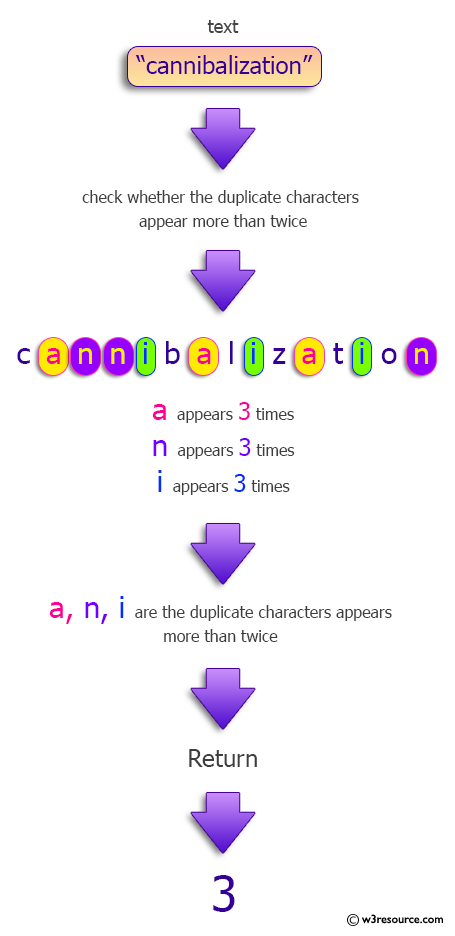
Sample Data:
(“abcdaa”) -> 1
("Tergiversation") ->0
Sample Solution-1:
Java Code:
public class Main {
public static void main(String[] args) {
// Define a string 'text' with certain characters
String text = "abcdaa";
System.out.println("Original String: " + text); // Display the original string
// Count and display the number of duplicate characters occurring more than twice in the string
System.out.println("Number of duplicate characters in the said String (Occurs more than twice.): " + test(text));
// Change the value of 'text' to another string
text = "Tergiversation";
System.out.println("\nOriginal String: " + text); // Display the original string
// Count and display the number of duplicate characters occurring more than twice in the string
System.out.println("Number of duplicate characters in the said String (Occurs more than twice.): " + test(text));
}
// Method to count duplicate characters occurring more than twice in a string
public static int test(String text) {
int ctr = 0; // Counter to store the count of duplicate characters
// Continue looping until the length of 'text' becomes zero
while (text.length() > 0) {
// Check if the count of a character (after removal) is more than 2 in the string
if (text.length() - text.replaceAll(text.charAt(0) + "", "").length() > 2) {
ctr++; // Increment the counter if duplicate characters are found more than twice
}
// Remove all occurrences of the first character from the string 'text'
text = text.replaceAll(text.charAt(0) + "", "");
}
return ctr; // Return the count of duplicate characters occurring more than twice
}
}
Sample Output:
Original String: abcdaa Number of duplicate characters in the said String (Occurs more than twice.): 1 Original String: Tergiversation Number of duplicate characters in the said String (Occurs more than twice.): 0
Flowchart:
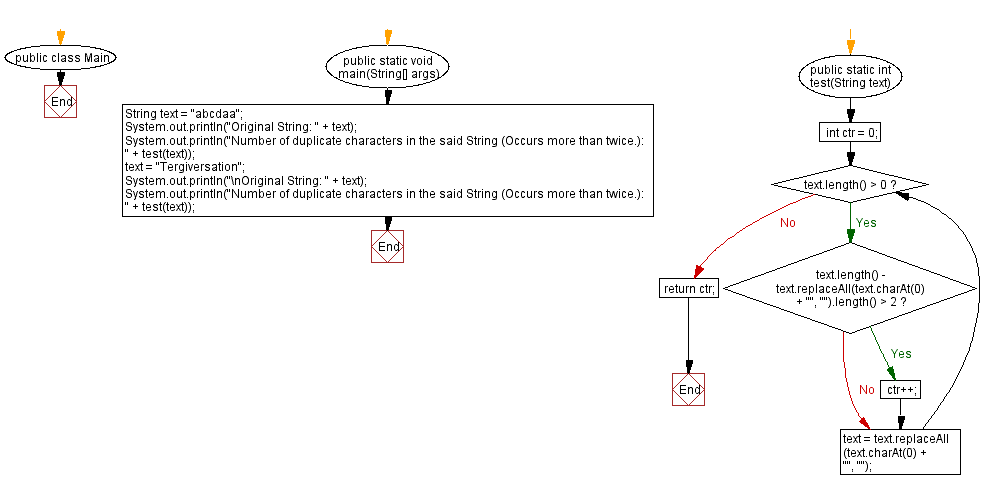
Sample Solution-2:
Java Code:
import java.util.function.Function;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
// Define a string 'text' with certain characters
String text = "abcdaa";
System.out.println("Original String: " + text); // Display the original string
// Count and display the number of duplicate characters occurring more than twice in the string
System.out.println("Number of duplicate characters in the said String (Occurs more than twice.): " + test(text));
// Change the value of 'text' to another string
text = "Tergiversation";
System.out.println("\nOriginal String: " + text); // Display the original string
// Count and display the number of duplicate characters occurring more than twice in the string
System.out.println("Number of duplicate characters in the said String (Occurs more than twice.): " + test(text));
}
// Method to count duplicate characters occurring more than twice in a string
public static int test(String text) {
return (int) text.chars() // Convert the string into an IntStream of characters
.boxed() // Box each integer value into its corresponding Integer object
.collect(Collectors.groupingBy(Function.identity(), Collectors.counting())) // Collect the characters into a map with their counts
.values() // Get the values (counts) from the map
.stream() // Convert the Collection into a Stream
.filter(ctr -> ctr > 2) // Filter the counts to keep those occurring more than twice
.count(); // Count the filtered occurrences
}
}
Sample Output:
Original String: abcdaa Number of duplicate characters in the said String (Occurs more than twice.): 1 Original String: Tergiversation Number of duplicate characters in the said String (Occurs more than twice.): 0
Flowchart:
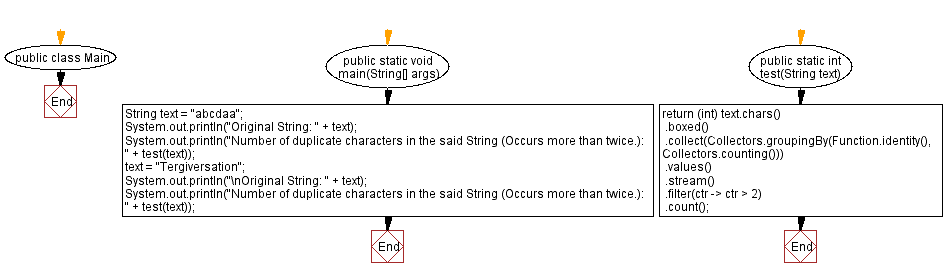
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous Java Exercise: Reverses the words in a string that have odd lengths.
Next Java Exercise: Remove a word from a given text.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics