Java: Count the occurrences of a given string in another given string
Write a Java program to count the occurrences of a given string in another given string.
Visual Presentation:
Sample Solution:
Java Code:
// Importing Arrays class from java.util package
import java.util.Arrays;
// Main class declaration
public class Main {
// Main method, entry point of the program
public static void main(String[] args) {
// Define main string and sub string
String main_string = "abcd abc aabc baa abcaa";
String sub_string = "aa";
// Count occurrences of sub string in main string using method count_sub_str_in_main_str
int countV1 = count_sub_str_in_main_str(main_string, sub_string);
// Print the count of occurrences of sub string in main string
System.out.println(sub_string + "' has occured " + countV1 + " times in '" + main_string + "'");
}
// Method to count occurrences of sub string in main string
public static int count_sub_str_in_main_str(String main_string, String sub_string) {
// Check for null strings
if (main_string == null || sub_string == null) {
throw new IllegalArgumentException("The given strings cannot be null");
}
// Check for empty strings
if (main_string.isEmpty() || sub_string.isEmpty()) {
return 0;
}
// Initialize variables for position and counter
int position = 0;
int ctr = 0;
int n = sub_string.length();
// Loop to find occurrences of sub string in main string
while ((position = main_string.indexOf(sub_string, position)) != -1) {
position = position + n;
ctr++;
}
return ctr;
}
}
Sample Output:
aa' has occured 3 times in 'abcd abc aabc baa abcaa'
Flowchart:
Count string in another string.
Main.java Code:
//MIT License: https://bit.ly/35gZLa3
import java.time.Clock;
import java.util.concurrent.TimeUnit;
public class Main {
private static final String STRING = "111111";
private static final String SUBSTRING = "11";
public static void main(String[] args) {
Clock clock = Clock.systemUTC();
long startTimeV1 = clock.millis();
int countV1 = Strings.countStringInStringV1(STRING, SUBSTRING);
displayExecutionTime(clock.millis() - startTimeV1);
System.out.println("V1: '" + SUBSTRING + "' has occured " + countV1 + " times in '" + STRING + "'");
long startTimeV2 = clock.millis();
int countV2 = Strings.countStringInStringV2(STRING, SUBSTRING);
displayExecutionTime(clock.millis() - startTimeV2);
System.out.println("V2: '" + SUBSTRING + "' has occured " + countV2 + " times in '" + STRING + "'");
long startTimeV3 = clock.millis();
int countV3 = Strings.countStringInStringV3(STRING, SUBSTRING);
displayExecutionTime(clock.millis() - startTimeV3);
System.out.println("V3: '" + SUBSTRING + "' has occured " + countV3 + " times in '" + STRING + "'");
}
private static void displayExecutionTime(long time) {
System.out.println("Execution time: " + time + " ms" + " ("
+ TimeUnit.SECONDS.convert(time, TimeUnit.MILLISECONDS) + " s)");
}
}
Strings.java Code:
//MIT License: https://bit.ly/35gZLa3
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public final class Strings {
private Strings() {
throw new AssertionError("Cannot be instantiated");
}
public static int countStringInStringV1(String string, String toFind) {
if (string == null || toFind == null) {
throw new IllegalArgumentException("The given strings cannot be null");
}
if (string.isEmpty() || toFind.isEmpty()) {
return 0;
}
int position = 0;
int count = 0;
int n = toFind.length();
while ((position = string.indexOf(toFind, position)) != -1) {
position = position + n;
count++;
}
return count;
}
public static int countStringInStringV2(String string, String toFind) {
if (string == null || toFind == null) {
throw new IllegalArgumentException("The given strings cannot be null");
}
if (string.isEmpty() || toFind.isEmpty()) {
return 0;
}
return string.split(Pattern.quote(toFind), -1).length - 1;
}
public static int countStringInStringV3(String string, String toFind) {
if (string == null || toFind == null) {
throw new IllegalArgumentException("The given strings cannot be null");
}
if (string.isEmpty() || toFind.isEmpty()) {
return 0;
}
Pattern pattern = Pattern.compile(Pattern.quote(toFind));
Matcher matcher = pattern.matcher(string);
int position = 0;
int count = 0;
while (matcher.find(position)) {
position = matcher.start() + 1;
count++;
}
return count;
}
}
Sample Output:
Execution time: 1 ms (0 s) V1: '11' has occured 3 times in '111111' Execution time: 2 ms (0 s) V2: '11' has occured 3 times in '111111' Execution time: 1 ms (0 s) V3: '11' has occured 5 times in '111111'
Flowchart:
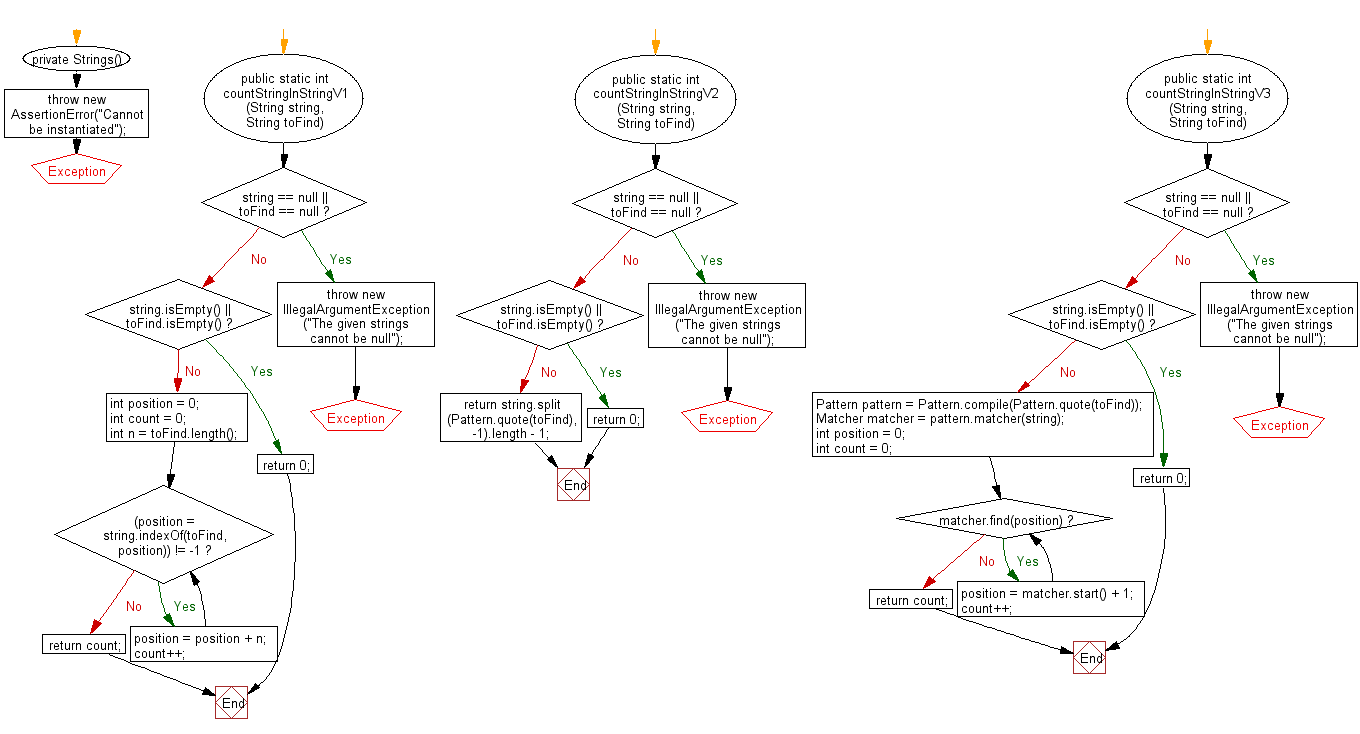
For more Practice: Solve these Related Problems:
- Write a Java program to count how many times a target substring appears in a main string using indexOf.
- Write a Java program to find overlapping occurrences of a substring in a string and return the count.
- Write a Java program to tally the frequency of a substring within a string using iterative search.
- Write a Java program to compute the number of times a specified substring appears in another string using regular expressions.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to sort in ascending and descending order by length of the given array of strings.
Next: Write a Java program to concatenate a given string with itself of a given number of times.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.