Java: Remove a specified character from a given string
Java String: Exercise-103 with Solution
Write a Java program to remove a specified character from a given string.
Visual Presentation:
Sample Solution:
Java Code:
// Define a class named Main
public class Main {
// The main method, entry point of the program
public static void main(String[] args) {
// Define the initial string and character to remove
String str1 = "abcdefabcdeabcdaaa";
char g_char = 'a';
// Remove the given character from the string
String result = remove_Character(str1, g_char);
// Print the original string
System.out.println("\nOriginal string:");
System.out.println(str1);
// Print the modified string after removing the character
System.out.println("\nSecond string:");
System.out.println(result);
}
// Method to remove a specific character from a string
public static String remove_Character(String str1, char g_char) {
// Check for null or empty input string
if (str1 == null || str1.isEmpty()) {
return "";
}
// Use StringBuilder to efficiently manipulate strings
StringBuilder sb = new StringBuilder();
// Convert input string to a character array for iteration
char[] chArray = str1.toCharArray();
// Iterate through the characters in the array
for (int i = 0; i < chArray.length; i++) {
// Append characters other than the given character to the StringBuilder
if (chArray[i] != g_char) {
sb.append(chArray[i]);
}
}
// Convert StringBuilder to String and return the modified string
return sb.toString();
}
}
Sample Output:
Original string: abcdefabcdeabcdaaa Second string: bcdefbcdebcd
Flowchart:
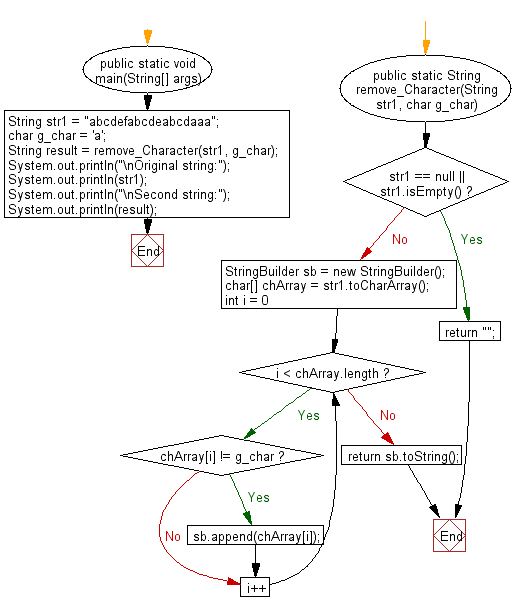
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to convert a given String to int, long, float and double.
Next: Write a Java program to sort in ascending and descending order by length of the given array of strings..
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics