Java: Get the character at the given index within the String
Java String: Exercise-1 with Solution
Write a Java program to get the character at the given index within the string.
Visualal Presentation:
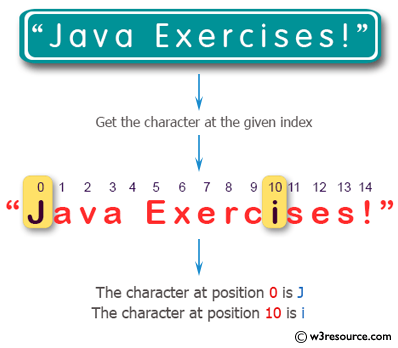
Sample Solution:
Java Code:
// Define a public class named Exercise1.
public class Exercise1 {
// Define the main method.
public static void main(String[] args) {
// Declare and initialize a string variable "str" with the value "Java Exercises!".
String str = "Java Exercises!";
// Print the original string.
System.out.println("Original String = " + str);
// Get the character at positions 0 and 10.
int index1 = str.charAt(0); // Get the ASCII value of the character at position 0.
int index2 = str.charAt(10); // Get the ASCII value of the character at position 10.
// Print out the results.
System.out.println("The character at position 0 is " +
(char)index1); // Print the character at position 0 by converting ASCII value to char.
System.out.println("The character at position 10 is " +
(char)index2); // Print the character at position 10 by converting ASCII value to char.
}
}
Sample Output:
Original String = Java Exercises! The character at position 0 is J The character at position 10 is i
Flowchart:
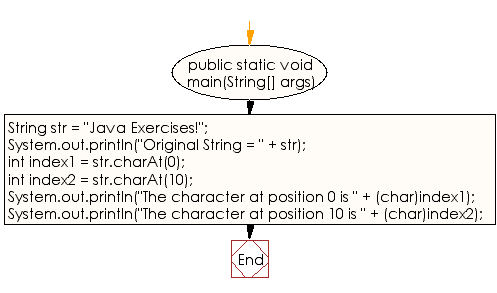
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java string Exercises.
Next: Write a Java program to get the character at the given index within the String.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/string/java-string-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics