Java Program: Remove Duplicate Elements from List using Streams
Write a Java program to remove all duplicate elements from a list using streams.
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
List < Integer > nums = Arrays.asList(10, 23, 22, 23, 24, 24, 33, 15, 26, 15);
System.out.println("Original List of numbers: " + nums);
// Remove duplicates
List < Integer > distinctNumbers = nums.stream()
.distinct()
.collect(Collectors.toList());
System.out.println("After removing duplicates from the said list: " + distinctNumbers);
}
}
Sample Output:
Original List of numbers: [10, 23, 22, 23, 24, 24, 33, 15, 26, 15] After removing duplicates from the said list: [10, 23, 22, 24, 33, 15, 26]
Explanation:
In the above exercise,
- The list of numbers is defined as nums and initialized with the Arrays.asList method.
- The distinct method is applied to the stream of numbers using the stream method on the nums list.
- This operation removes duplicate elements from the stream.
- The resulting stream is collected back into a list using the collect method with Collectors.toList().
Flowchart:
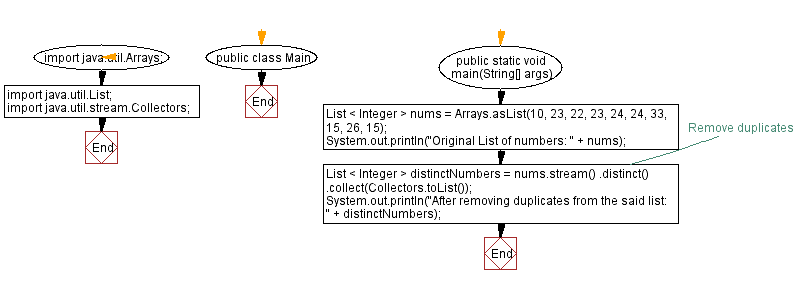
For more Practice: Solve these Related Problems:
- Write a Java program to remove duplicate integers from a list using streams and then sort the unique values in ascending order.
- Write a Java program to remove duplicate strings from a list using streams while ignoring case sensitivity.
- Write a Java program to remove duplicate elements from a list using streams and then count the total number of unique elements.
- Write a Java program to remove duplicates from a list of objects using streams based on a specified attribute.
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Sum of Even and Odd Numbers in a List using Streams.
Next: Count Strings Starting with Specific Letter using Streams.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics