Java: Find a specified element in a given array of elements using Linear Search
Java Search: Exercise-2 with Solution
Write a Java program to find a specified element in a given array of elements using Linear Search.
Sample Solution:
Java Code:
public class Main {
static int [] nums;
public static void main(String[] args) {
nums = new int[]{3,2,4,5,6,6,7,8,9,9,0,9};
int result = Linear_Search(nums, 6);
if(result == -1)
{
System.out.print("Not present in the array!");
}
else
System.out.print("Number found at index "+result);
}
private static int Linear_Search(int [] nums,int search)
{
for(int i=0;i<nums.length;i++)
{
if(nums[i]==search)
{
return i;
}
}
return -1;
}
}
Sample Output:
Number found at index 4
Flowchart:
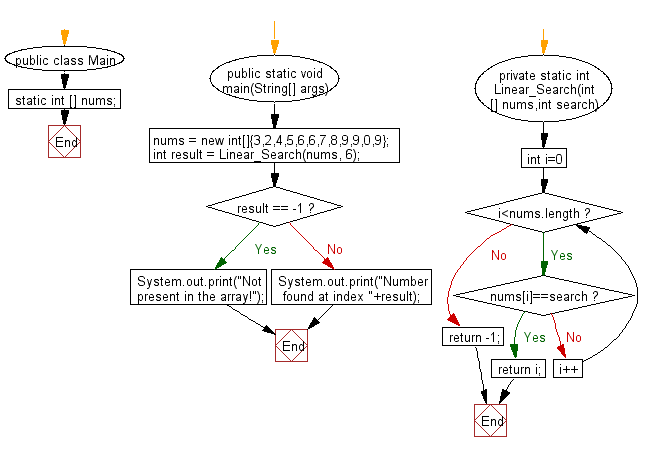
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to find a specified element in a given array of elements using Binary Search.
Next: Write a Java program to find a specified element in a given sorted array of elements using Jump Search.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics