Java Recursive Method: Calculate the nth Fibonacci number
Recursive Nth Fibonacci Number
Write a Java recursive method to calculate the nth Fibonacci number.
Sample Solution:
Java Code:
public class FibonacciCalculator {
public static int calculateFibonacci(int n) {
// Base case: Fibonacci numbers at positions 0 and 1 are 0 and 1, respectively
if (n == 0) {
return 0;
} else if (n == 1) {
return 1;
}
// Recursive case: sum of the previous two Fibonacci numbers
return calculateFibonacci(n - 1) + calculateFibonacci(n - 2);
}
public static void main(String[] args) {
int position = 0;
int fibonacciNumber = calculateFibonacci(position);
System.out.println("The Fibonacci number at position " + position + " is: " + fibonacciNumber);
position = 3;
fibonacciNumber = calculateFibonacci(position);
System.out.println("\nThe Fibonacci number at position " + position + " is: " + fibonacciNumber);
position = 9;
fibonacciNumber = calculateFibonacci(position);
System.out.println("\nThe Fibonacci number at position " + position + " is: " + fibonacciNumber);
}
}
Sample Output:
The Fibonacci number at position 0 is: 0 The Fibonacci number at position 3 is: 2 The Fibonacci number at position 9 is: 34
Explanation:
In the above exercises -
The "calculateFibonacci()" method follows the recursive definition of the Fibonacci sequence. It has two cases:
- case 1: If n is 0, it returns 0.
- case 2: If n is 1, it returns 1.
These are the termination conditions for recursion.
For any positive n greater than 1, the method recursively calculates the Fibonacci number by summing the previous two Fibonacci numbers (calculated using the same method). This process is repeated until n reaches one of the base cases.
In the main() method, we demonstrate the calculateFibonacci() method by calculating the Fibonacci number at position 8 and printing the result.
Flowchart:
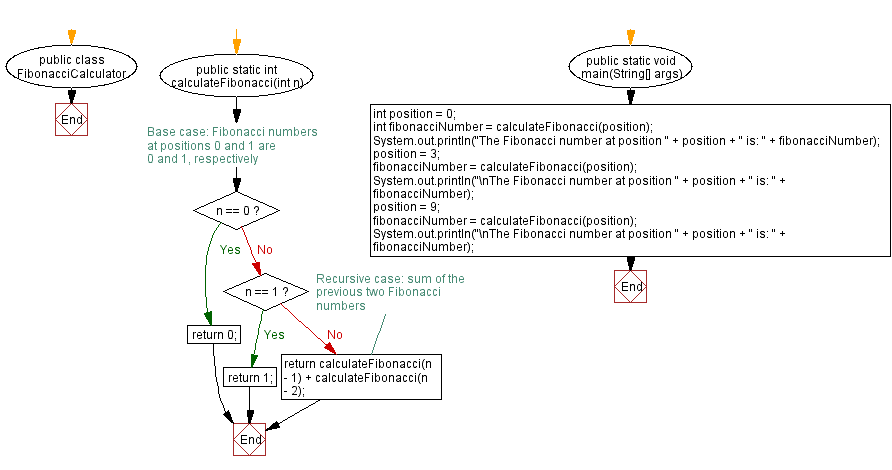
For more Practice: Solve these Related Problems:
- Write a Java program to compute the nth Fibonacci number recursively using memoization to optimize performance.
- Write a Java program to calculate the nth Fibonacci number using tail recursion.
- Write a Java program to recursively generate and print the first n Fibonacci numbers.
- Write a Java program to compute the nth Fibonacci number recursively while counting and displaying the number of recursive calls.
Java Code Editor:
Java Recursive Previous: Calculate the sum of numbers from 1 to n.
Java Recursive Next: String palindrome detection.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.