Java recursive array sorting: Check ascending order
Java Recursive: Exercise-15 with Solution
Write a Java recursive method to check if a given array is sorted in ascending order.
Sample Solution:
Java Code:
import java.util.Arrays;
public class ArraySortChecker {
public static boolean isSorted(int[] arr) {
return isSorted(arr, 0);
}
private static boolean isSorted(int[] arr, int index) {
// Base case: if the index reaches the end of the array,
// the array is sorted
if (index == arr.length - 1) {
return true;
}
// Recursive case: check if the element at the current index
// is greater than the next element,
// and recursively call the method with the next index
if (arr[index] > arr[index + 1]) {
return false;
}
return isSorted(arr, index + 1);
}
public static void main(String[] args) {
int[] array1 = {
1,
2,
3,
4,
5
};
System.out.println("Original Array: " + Arrays.toString(array1));
boolean sorted1 = isSorted(array1);
System.out.println("Is array1 sorted in ascending order? " + sorted1); // Output: true
int[] array2 = {
4,
5,
3,
2,
1
};
System.out.println("Original Array: " + Arrays.toString(array2));
boolean sorted2 = isSorted(array2);
System.out.println("Is array2 sorted in ascending order? " + sorted2); // Output: false
}
}
Sample Output:
Original Array: [1, 2, 3, 4, 5] Is array1 sorted in ascending order? true Original Array: [4, 5, 3, 2, 1] Is array2 sorted in ascending order? false
Explanation:
In the above exercises -
First, we define a class "ArraySortChecker" that includes a recursive method isSorted() to check if a given array arr is sorted in ascending order.
The isSorted() method has two cases:
- Base case: If the index reaches the end of the array (index == arr.length - 1), we have traversed the entire array and return true to indicate that the array is sorted.
- Recursive case: For any index that is within the bounds of the array, we check if the element at that index is greater than the next element. If it is, we return false to indicate that the array is not sorted. Otherwise, we recursively call the method with the next index.
In the main() method, we demonstrate the usage of the isSorted() method by checking if two arrays (array1 and array2) are sorted in ascending order and printing the results.
Flowchart:
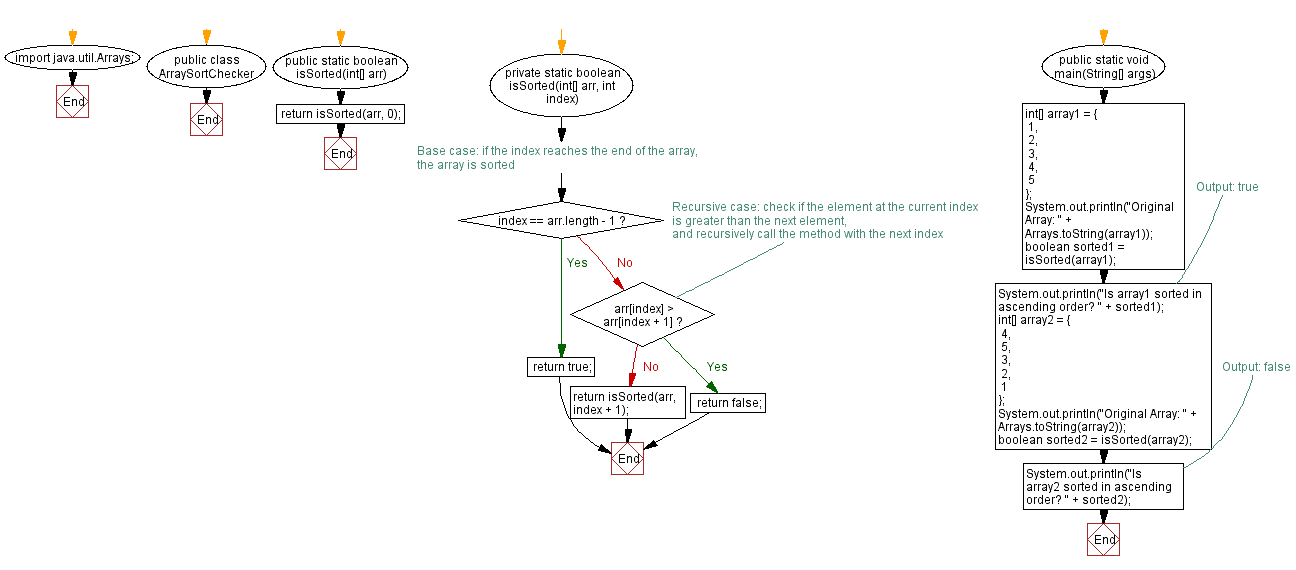
Java Code Editor:
Java Recursive Previous: Find the sum of digits in an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/recursive/java-recursive-exercise-15.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics