Java: Check a number is a cube or not
Java Numbers: Exercise-18 with Solution
Write a Java program to check if a number is a cube or not.
In arithmetic and algebra, the cube of a number n is its third power: the result of the number multiplied by itself twice:
n3 = n × n × n
Input Data:
Input a number: 8
Pictorial Presentation:
Sample Solution:
Java Code:
import java.util.Scanner;
public class Example18 {
public static void main( String args[] ){
Scanner sc = new Scanner( System.in );
System.out.print("Input a number: ");
int num = sc.nextInt();
int n = (int) Math.round(Math.pow(num, 1.0/3.0));
if((num == n * n * n))
{
System.out.print("Number is a cube.");
}
else
{
System.out.print("Number is not a cube.");
}
System.out.println("\n");
}
}
Sample Output:
Input a number: 8 Number is a cube.
Flowchart:
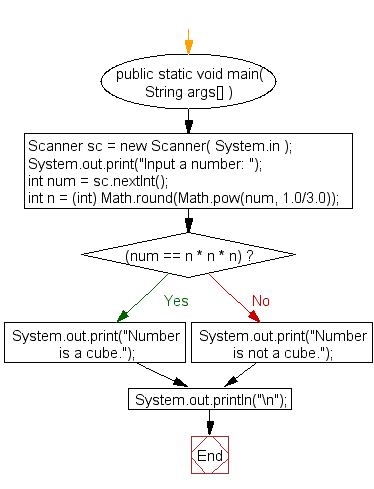
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to check if a given number is circular prime or not.
Next: Write a Java program to check a number is a cyclic or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/numbers/java-number-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics