Java: Display the middle character of a string
Java Method: Exercise-3 with Solution
Write a Java method to display the middle character of a string.
Note: a) If the length of the string is odd there will be two middle characters.
b) If the length of the string is even there will be one middle character.
Test Data:
Input a string: 350
Pictorial Presentation:
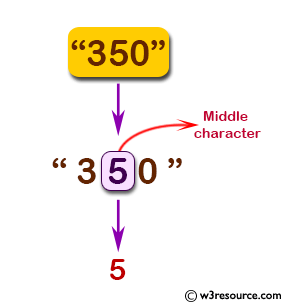
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise3 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input a string: ");
String str = in.nextLine();
System.out.print("The middle character in the string: " + middle(str)+"\n");
}
public static String middle(String str)
{
int position;
int length;
if (str.length() % 2 == 0)
{
position = str.length() / 2 - 1;
length = 2;
}
else
{
position = str.length() / 2;
length = 1;
}
return str.substring(position, position + length);
}
}
Sample Output:
Input a string: 350 The middle character in the string: 5
Flowchart:
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java method to compute the average of three numbers.
Next: Write a Java method to count all vowels in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics