Java: Create the area of a pentagon
Calculate Pentagon Area
Write a Java method to create a pentagon's area.
Pictorial Presentation:
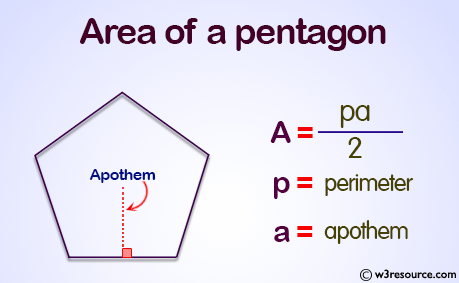
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise14 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Input the number of sides: ");
int n = input.nextInt();
System.out.print("Input the side: ");
double side = input.nextDouble();
System.out.println("The area of the pentagon is " + pentagon_area(n, side));
}
public static double pentagon_area(int n, double s) {
return (n * s * s) / (4 * Math.tan(Math.PI/n));
}
}
Sample Output:
Input the number of sides: 5 Input the side: 6 The area of the pentagon is 61.93718642120281
Flowchart:
For more Practice: Solve these Related Problems:
- Write a Java program to calculate the area of a regular pentagon using its side length and apothem.
- Write a Java program to compute the area of a pentagon by dividing it into five triangles and summing their areas.
- Write a Java program to determine the area of a pentagon given its perimeter and apothem.
- Write a Java program to calculate the area of an irregular pentagon given the coordinates of its vertices.
Go to:
PREV : Calculate Triangle Area.
NEXT : Display Current Date and Time.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.