Java: Check whether a string is a valid password
Validate Password
Write a Java method to check whether a string is a valid password.
Password rules:
A password must have at least ten characters.
A password consists of only letters and digits.
A password must contain at least two digits.
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise11 {
public static final int PASSWORD_LENGTH = 8;
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print(
"1. A password must have at least eight characters.\n" +
"2. A password consists of only letters and digits.\n" +
"3. A password must contain at least two digits \n" +
"Input a password (You are agreeing to the above Terms and Conditions.): ");
String s = input.nextLine();
if (is_Valid_Password(s)) {
System.out.println("Password is valid: " + s);
} else {
System.out.println("Not a valid password: " + s);
}
}
public static boolean is_Valid_Password(String password) {
if (password.length() < PASSWORD_LENGTH) return false;
int charCount = 0;
int numCount = 0;
for (int i = 0; i < password.length(); i++) {
char ch = password.charAt(i);
if (is_Numeric(ch)) numCount++;
else if (is_Letter(ch)) charCount++;
else return false;
}
return (charCount >= 2 && numCount >= 2);
}
public static boolean is_Letter(char ch) {
ch = Character.toUpperCase(ch);
return (ch >= 'A' && ch <= 'Z');
}
public static boolean is_Numeric(char ch) {
return (ch >= '0' && ch <= '9');
}
}
Sample Output:
1. A password must have at least eight characters. 2. A password consists of only letters and digits. 3. A password must contain at least two digits Input a password (You are agreeing to the above Terms and Conditions.): abcd1234 Password is valid: abcd1234
Flowchart:
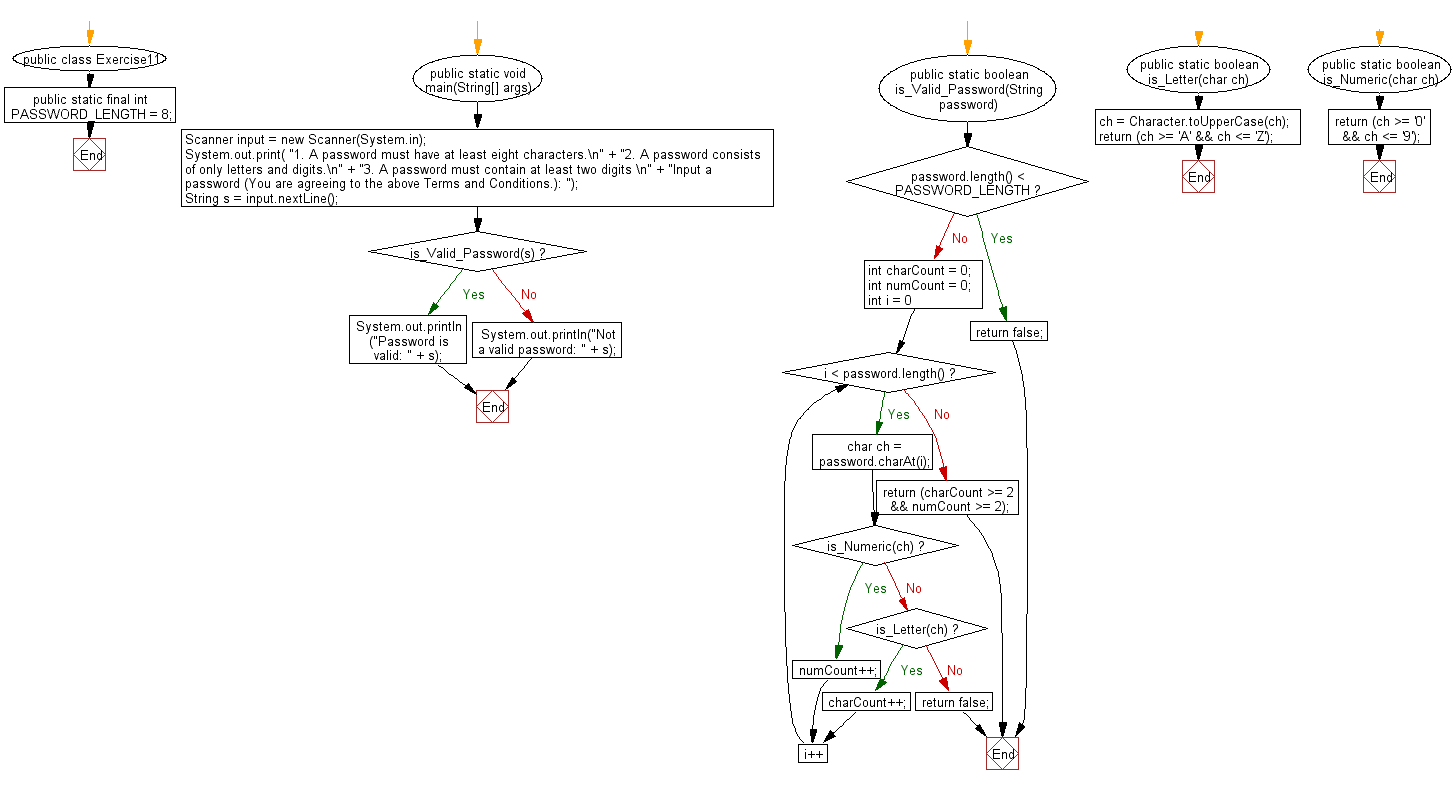
For more Practice: Solve these Related Problems:
- Write a Java program to validate a password that must contain at least one special character in addition to letters and digits.
- Write a Java program to assess password strength by checking for uppercase, lowercase, digits, and symbols.
- Write a Java program to validate a password using regular expressions and provide specific error messages for each rule violation.
- Write a Java program to check if a password is valid when it must start with a letter and contain no whitespace.
Go to:
PREV : Check Leap Year.
NEXT : Display n-by-n Matrix.
Java Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.