Java: Convert a float value to absolute value
Java Math Exercises: Exercise-9 with Solution
Write a Java program to convert a floating value to an absolute value.
Sample Solution:
Java Code:
import java.util.*;
public class Example9 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input a float number: ");
float x = in.nextFloat();
System.out.printf("The absolute value of %.2f is: %.2f",x, convert(x));
System.out.printf("\n");
}
public static float convert(float n)
{
float absvalue = (n >= 0) ? n : -n;
return absvalue;
}
}
Sample Output:
Input a float number: 12.53 The absolute value of 12.53 is: 12.53
Flowchart:
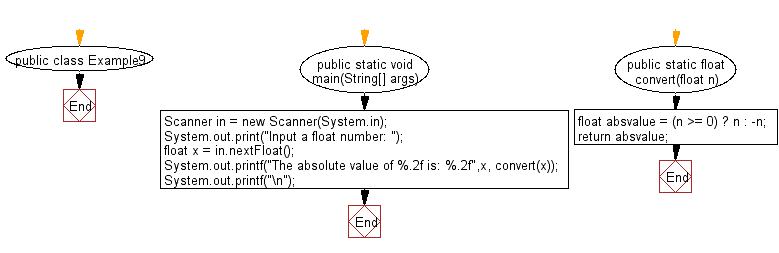
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to convert an integer value to absolute value.
Next: Write a Java program to accept a float value of number and return a rounded float value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/math/java-math-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics