Java Program: Lambda expression for checking uppercase, lowercase, or mixedcase strings
22. Check case of strings in list using lambda
Write a Java program to implement a lambda expression to check if a list of strings are all uppercase or all lowercase or mixedcase.
Sample Solution:
Java Code:
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
import java.util.function.Function;
public class Main {
public static void main(String[] args) {
List < String > strings = Arrays.asList("Java", "JAVA", "java");
System.out.println("Array elements: " + strings);
// Check if the list is all uppercase using lambda expression
boolean isAllUppercase = checkCase(strings, s -> s.equals(s.toUpperCase()), String::toUpperCase);
System.out.println("Is all uppercase? " + isAllUppercase);
// Check if the list is all lowercase using lambda expression
boolean isAllLowercase = checkCase(strings, s -> s.equals(s.toLowerCase()), String::toLowerCase);
System.out.println("Is all lowercase? " + isAllLowercase);
// Check if the list is mixed case
boolean isMixedCase = !isAllUppercase && !isAllLowercase;
System.out.println("Is mixed case? " + isMixedCase);
List < String > strings1 = Arrays.asList("JAVA", "PYTHON", "ABC");
System.out.println("\nArray elements: " + strings1);
// Check if the list is all uppercase using lambda expression
isAllUppercase = checkCase(strings1, s -> s.equals(s.toUpperCase()), String::toUpperCase);
System.out.println("Is all uppercase? " + isAllUppercase);
// Check if the list is all lowercase using lambda expression
isAllLowercase = checkCase(strings1, s -> s.equals(s.toLowerCase()), String::toLowerCase);
System.out.println("Is all lowercase? " + isAllLowercase);
// Check if the list is mixed case
isMixedCase = !isAllUppercase && !isAllLowercase;
System.out.println("Is mixed case? " + isMixedCase);
List < String > strings2 = Arrays.asList("java");
System.out.println("\nArray elements: " + strings2);
// Check if the list is all uppercase using lambda expression
isAllUppercase = checkCase(strings2, s -> s.equals(s.toUpperCase()), String::toUpperCase);
System.out.println("Is all uppercase? " + isAllUppercase);
// Check if the list is all lowercase using lambda expression
isAllLowercase = checkCase(strings2, s -> s.equals(s.toLowerCase()), String::toLowerCase);
System.out.println("Is all lowercase? " + isAllLowercase);
// Check if the list is mixed case
isMixedCase = !isAllUppercase && !isAllLowercase;
System.out.println("Is mixed case? " + isMixedCase);
}
public static boolean checkCase(List < String > strings, Predicate < String > checkFunction, Function < String, String > convertFunction) {
String firstString = strings.get(0);
String convertedString = convertFunction.apply(firstString);
return strings.stream()
.allMatch(s -> checkFunction.test(s));
}
}
Sample Output:
Array elements: [Java, JAVA, java] Is all uppercase? false Is all lowercase? false Is mixed case? true Array elements: [JAVA, PYTHON, ABC] Is all uppercase? true Is all lowercase? false Is mixed case? false Array elements: [java] Is all uppercase? false Is all lowercase? true Is mixed case? false
Explanation:
In the above exercise -
The main() method:
- Creates a list of strings named strings.
- Calls the checkCase method to perform case checks and prints the results.
The checkCase() method:
- Takes three parameters: the list of strings to check, a Predicate<String> to define the case check condition, and a Function<String, String> to convert the first string for comparison.
- Retrieves the first string from the list using strings.get(0) and assigns it to firstString.
- Apply the conversion function convertFunction to firstString and assign the result to convertedString.
- Use the stream() method in the strings list to create a stream of strings.
- Apply the allMatch intermediate operation on the stream and provide a lambda expression as the condition to check if all strings satisfy the given predicate checkFunction.
- Returns the result of the allMatch operation, which indicates whether all strings in the list pass the case check.
Flowchart:
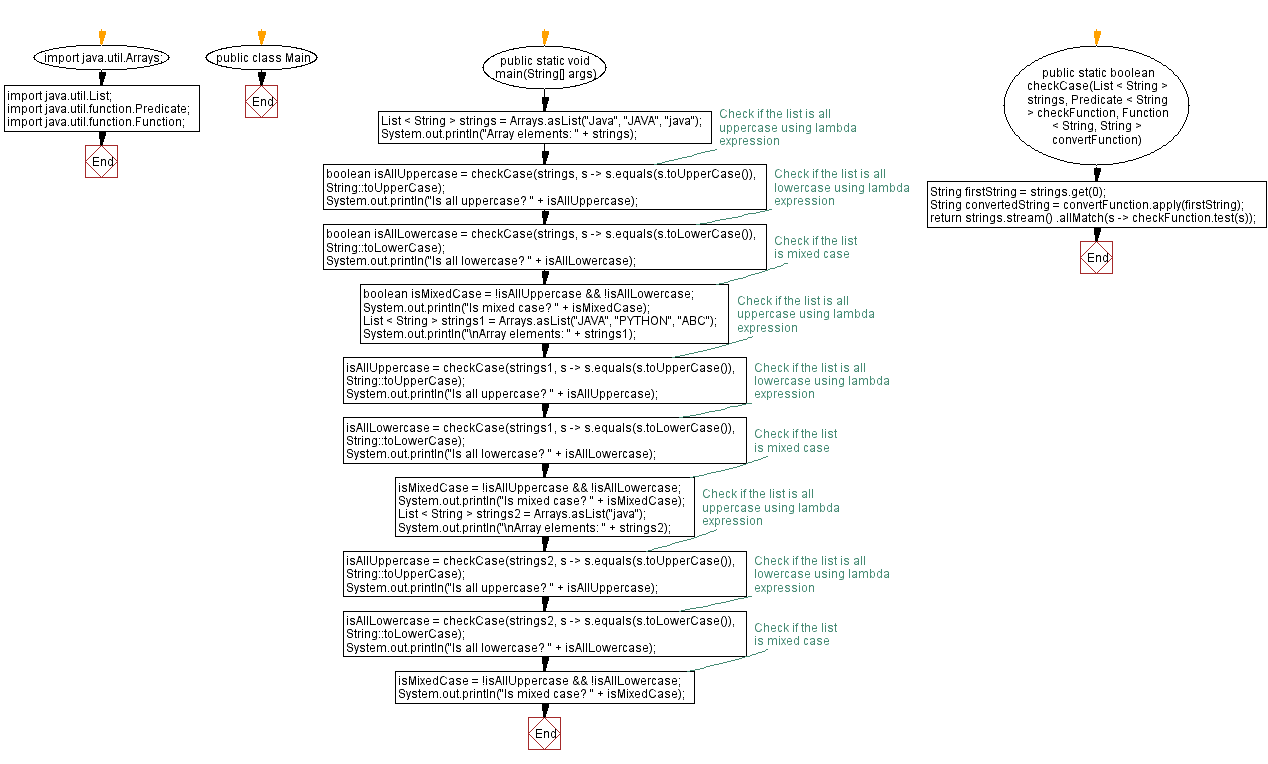
For more Practice: Solve these Related Problems:
- Write a Java program to implement a lambda expression that checks if every string in a list is uppercase using allMatch().
- Write a Java program to create a lambda that validates whether all strings in a list are lowercase and returns a boolean result.
- Write a Java program to implement a lambda expression that determines if a list of strings is mixed-case by comparing counts of uppercase and lowercase letters.
- Write a Java program to chain lambda expressions to group strings by their case type and then check for uniformity.
Go to:
Live Demo:
Java Code Editor:
Improve this sample solution and post your code through Disqus
PREV : Sum all primes in range using lambda.
NEXT : Find average string length using lambda.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.