JavaFX Country selection application
Write a JavaFX application that builds a country selection form using a ChoiceBox or ComboBox. Populate the dropdown with a list of countries, and when a country is selected, display its capital in a label.
Sample Solution:
JavaFx Code:
In the above JavaFX application, we use a ComboBox to select a country. A map is used to store the capitals of each country. When a country is selected from the ComboBox, it retrieves the capital from the map and displays it in a label. The elements are organized using a 'VBox'.
Sample Output:
Flowchart:
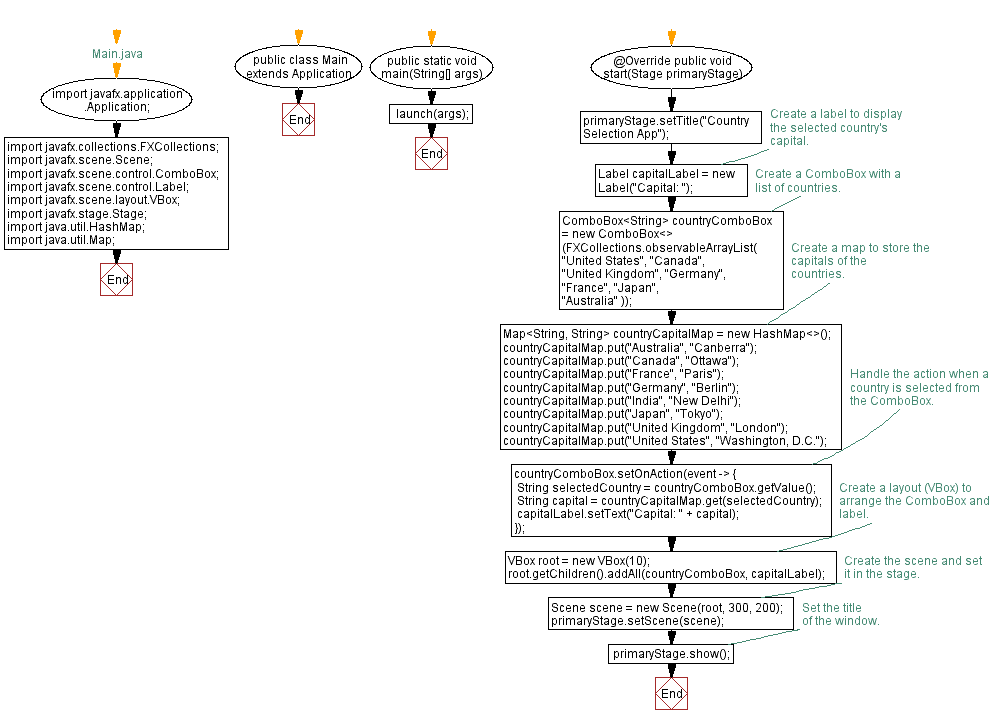
Java Code Editor:
Previous: JavaFX Month days calculator.
Next: JavaFX CheckBox selection.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics