JavaFX Chat application with ListView
21. Chat App with ListView and TextArea
Write a JavaFX program to build a chat application with a ListView for displaying messages. Allow users to enter and send messages using a TextArea. Messages should be added to the ListView when sent.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ListView;
import javafx.scene.control.TextArea;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
private ObservableList messages;
private ListView messageListView;
private TextArea messageTextArea;
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Chat Application");
messages = FXCollections.observableArrayList();
messageListView = new ListView<>(messages);
messageTextArea = new TextArea();
Button sendButton = new Button("Send");
sendButton.setOnAction(event -> {
String message = messageTextArea.getText();
if (!message.isEmpty()) {
messages.add("You: " + message);
messageTextArea.clear();
}
});
HBox inputBox = new HBox(10);
inputBox.getChildren().addAll(messageTextArea, sendButton);
VBox root = new VBox(10);
root.getChildren().addAll(messageListView, inputBox);
Scene scene = new Scene(root, 400, 300);
primaryStage.setScene(scene);
primaryStage.show();
}
}
In the above JavaFX exercise, you can input a message in the TextArea and click the "Send" button to add your message to the ListView. Messages sent are displayed with "You: " as a prefix. This serves as a basic chat interface.
For a complete chat application, you'd need to implement networking or communication between multiple users and handle incoming messages.
Sample Output:
Flowchart:
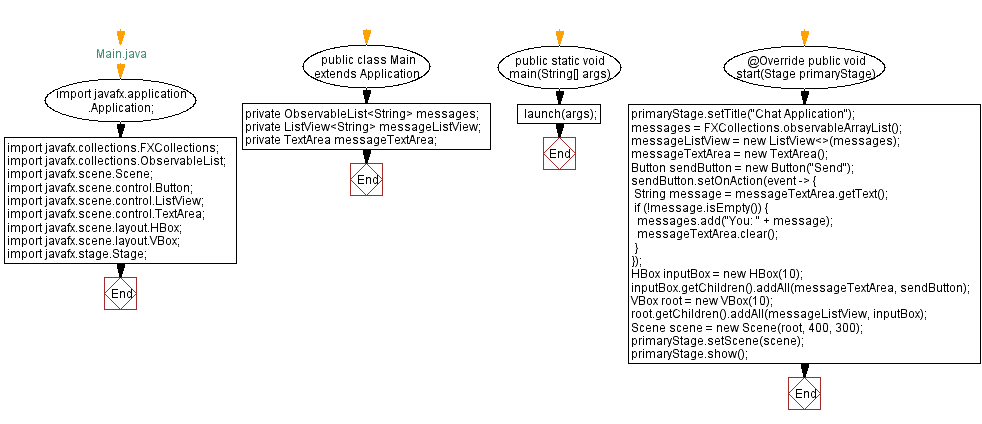
Go to:
PREV : To-Do List App with ListView.
NEXT : JavaFX Layout Management Exercises Home.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.