JavaFX Download manager with ProgressBar
JavaFx User Interface Components: Exercise-18 with Solution
Write a JavaFX program to build a download manager application with a ProgressBar to show download progress. When a download is in progress, update the ProgressBar accordingly.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.concurrent.Task;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ProgressBar;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Download Manager");
ProgressBar progressBar = new ProgressBar(0);
Button startButton = new Button("Start Download");
startButton.setOnAction(event -> {
// Simulate a download task.
Task<Void> downloadTask = createDownloadTask(progressBar);
Thread downloadThread = new Thread(downloadTask);
downloadThread.start();
});
VBox root = new VBox(10);
root.getChildren().addAll(progressBar, startButton);
Scene scene = new Scene(root, 300, 100);
primaryStage.setScene(scene);
primaryStage.show();
}
private Task<Void> createDownloadTask(ProgressBar progressBar) {
Task<Void> downloadTask = new Task<Void>() {
@Override
protected Void call() {
for (int i = 0; i <= 100; i++) {
updateProgress(i, 100);
try {
Thread.sleep(100); // Simulate download delay.
} catch (InterruptedException e) {
// Handle interruption.
break;
}
}
return null;
}
};
progressBar.progressProperty().bind(downloadTask.progressProperty());
return downloadTask;
}
}
In the exercise above, when we click the "Start Download" button, it starts a simulated download task. The 'ProgressBar' updates to show progress. This is a simplified representation of a download manager. In a real download manager, you replace the simulated download with actual file downloads using libraries and handle network-related tasks properly.
Note: Remember that real download managers require robust error handling, support for multiple downloads, user authentication, and more features, which are beyond the scope of a simple example.
Sample Output:
Flowchart:
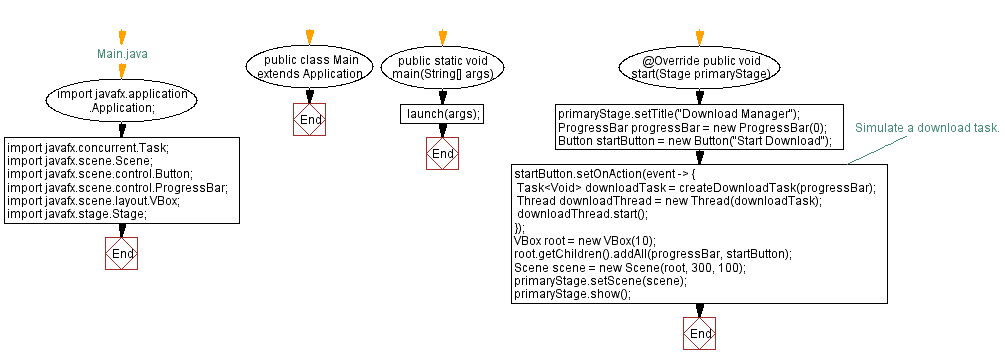
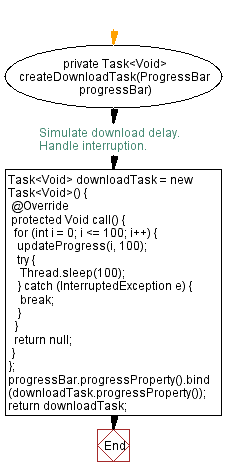
Java Code Editor:
Previous: JavaFX Media player interface with progress slider.
Next: JavaFX TextArea to ListView example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/javafx/javafx-user-interface-components-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics