JavaFX Media player interface with progress slider
17. Media Progress Slider Demo
Write a JavaFX media player interface with a slider to control playback progress. When the slider is moved, update the video or audio progress. Integration of media libraries is required to create a full-fledged JavaFX media player interface with video and audio playback. So create a simplified example that demonstrates how to implement a JavaFX interface with a slider to control progress. Use a dummy label to represent playback progress.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.beans.InvalidationListener;
import javafx.beans.Observable;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Slider;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Media Player");
// Create a label to represent playback progress.
Label progressLabel = new Label("Progress: 0%");
// Create a slider to control playback progress.
Slider progressSlider = new Slider(0, 100, 0);
progressSlider.setShowTickLabels(true);
progressSlider.setShowTickMarks(true);
// Bind the label text to the slider value for progress.
progressSlider.valueProperty().addListener(new InvalidationListener() {
@Override
public void invalidated(Observable observable) {
double value = progressSlider.getValue();
progressLabel.setText("Progress: " + String.format("%.1f%%", value));
}
});
// Create a layout (VBox) to arrange the components.
VBox root = new VBox(10);
root.getChildren().addAll(progressLabel, progressSlider);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 100);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.show();
}
}
The above exercise demonstrates a basic media player interface with a slider to control playback progress. In a real media player application, you would integrate a media library (such as JavaFX Media or third-party libraries like VLCJ or JavaZoom) to handle video or audio playback and update the slider based on media progress.
Sample Output:
Flowchart:
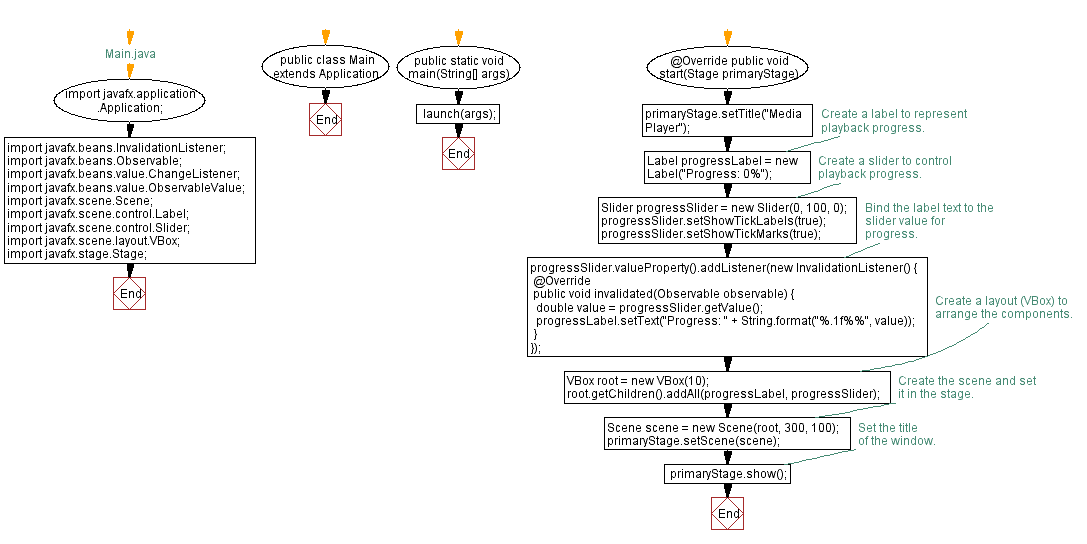
Go to:
PREV : Circle Radius Control with Slider.
NEXT : Download Progress with ProgressBar.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.