JavaFX wireless mode selection application
15. Wireless Mode Selector with ToggleButtons
Write a JavaFX application for choosing a wireless mode using ToggleButtons (e.g., "Bluetooth," "WiFi," "hot-spot"). Highlight the selected wireless mode.
Sample Solution:
JavaFx Code:
Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ToggleButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Wireless mode Selection");
// Create a ToggleGroup for the ToggleButtons.
ToggleGroup toggleGroup = new ToggleGroup();
// Create ToggleButtons for Wireless mode Selection.
ToggleButton bluetoothButton = createToggleButton("Bluetooth", toggleGroup);
ToggleButton wifiButton = createToggleButton("WiFi", toggleGroup);
ToggleButton hotspotButton = createToggleButton("Hotspot", toggleGroup);
// Create a layout (HBox) to arrange the ToggleButtons.
HBox root = new HBox(10);
root.getChildren().addAll(bluetoothButton, wifiButton, hotspotButton);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 100);
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm()); // Load CSS file
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.show();
}
// Create a ToggleButton and add it to the ToggleGroup.
private ToggleButton createToggleButton(String text, ToggleGroup toggleGroup) {
ToggleButton button = new ToggleButton(text);
button.setToggleGroup(toggleGroup);
return button;
}
}
styles.css
.toggle-button:selected {
-fx-background-color: lightblue;
}
In the above JavaFX application, we use ToggleButtons to choose between different difficulty levels (Bluetooth, WiFi, Hotspot). When a ToggleButton is selected, it is highlighted with a light blue background. The elements are organized using an 'HBox'.
Note: Place the "styles.css" file in the same directory as your Java source code. This stylesheet defines the background color for selected 'ToggleButtons'.
Sample Output:
Flowchart:
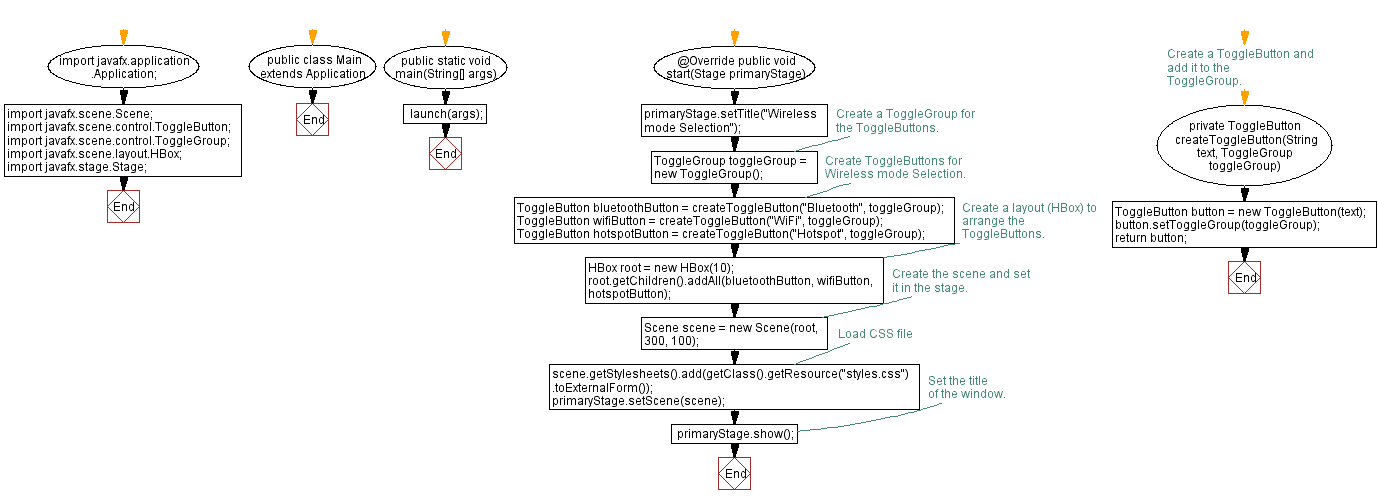
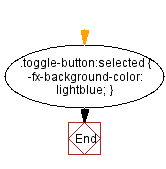
Go to:
PREV : ToggleButtons Color Preference.
NEXT : Circle Radius Control with Slider.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.