JavaFX ToggleButtons color preference form
Write a JavaFX preference form with ToggleButtons to choose a color (e.g., "Red," "Green"). Ensure only one color can be selected at a time and display the chosen color.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.ToggleButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Color Preference Form");
// Create a label to display the chosen color.
Label colorLabel = new Label("Chosen Color: None");
// Create a ToggleGroup for the ToggleButtons to allow only one selection.
ToggleGroup toggleGroup = new ToggleGroup();
// Create ToggleButtons for color options.
ToggleButton redButton = new ToggleButton("Red");
redButton.setToggleGroup(toggleGroup);
ToggleButton greenButton = new ToggleButton("Green");
greenButton.setToggleGroup(toggleGroup);
// Handle the action when a ToggleButton is selected.
redButton.setOnAction(event -> updateColorLabel(colorLabel, "Red"));
greenButton.setOnAction(event -> updateColorLabel(colorLabel, "Green"));
// Create a layout (VBox) to arrange the ToggleButtons and label.
VBox root = new VBox(10);
root.getChildren().addAll(redButton, greenButton, colorLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.show();
}
// Update the label to display the chosen color.
private void updateColorLabel(Label label, String chosenColor) {
label.setText("Chosen Color: " + chosenColor);
}
}
In the above JavaFX preference form, we use ToggleButtons to choose between Red and Green. A ToggleGroup ensures that only one color can be selected at a time, and the label displays the chosen color. The elements are organized using a 'VBox'.
Sample Output:
Flowchart:
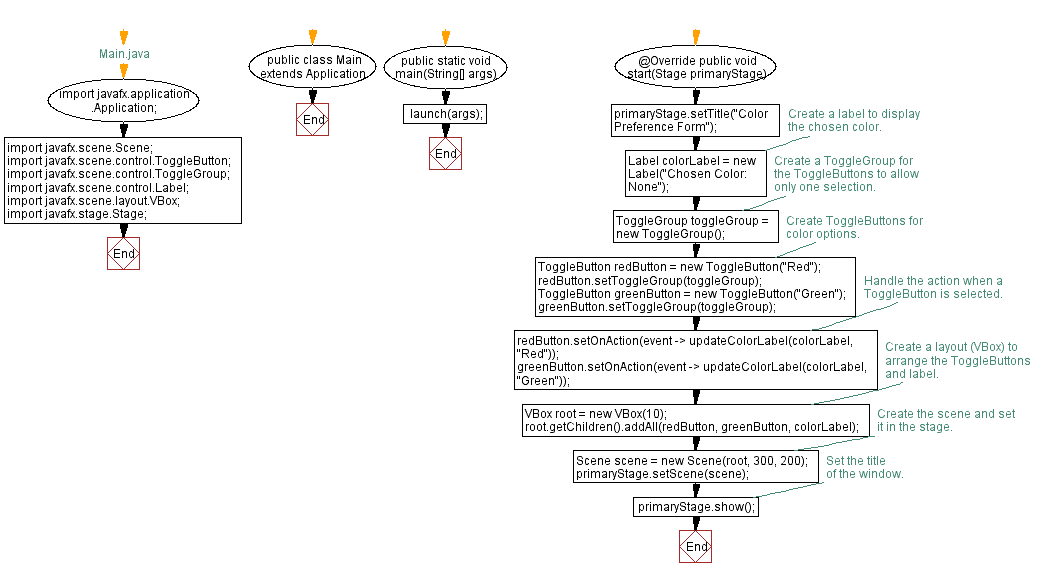
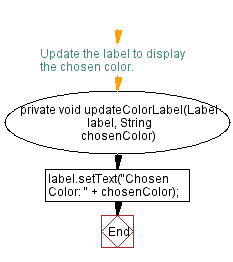
Java Code Editor:
Previous: JavaFX ToggleButton Radio Application.
Next: JavaFX wireless mode selection application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics