JavaFX preference form using RadioButton
11. RadioButton Boolean Selector
Write a JavaFX preference form using RadioButton options for choosing a Boolean value (e.g., "True," "False"). Display a message based on the selected value.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ToggleGroup;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Preference Form App");
// Create a label to display the selected Value.
Label themeLabel = new Label("Selected Value: ");
// Create a ToggleGroup for the RadioButton options.
ToggleGroup toggleGroup = new ToggleGroup();
// Create RadioButton options for "True" and "False."
RadioButton trueRadioButton = new RadioButton("True");
trueRadioButton.setToggleGroup(toggleGroup);
RadioButton falseRadioButton = new RadioButton("False");
falseRadioButton.setToggleGroup(toggleGroup);
// Handle the action when a RadioButton is selected.
trueRadioButton.setOnAction(event -> updateThemeLabel(themeLabel, true));
falseRadioButton.setOnAction(event -> updateThemeLabel(themeLabel, false));
// Create a layout (VBox) to arrange the RadioButton options and label.
VBox root = new VBox(10);
root.getChildren().addAll(trueRadioButton, falseRadioButton, themeLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.show();
}
// Update the label to display the selected Value.
private void updateThemeLabel(Label label, boolean isTrue) {
String selectedTheme = isTrue ? "True" : "False";
label.setText("Selected Value: " + selectedTheme);
}
}
In the above JavaFX application, we use RadioButton options for "True" and "False." When a RadioButton is selected, the label displays the selected theme ("True" or "False"). The elements are organized using a 'VBox'.
Sample Output:
Flowchart:
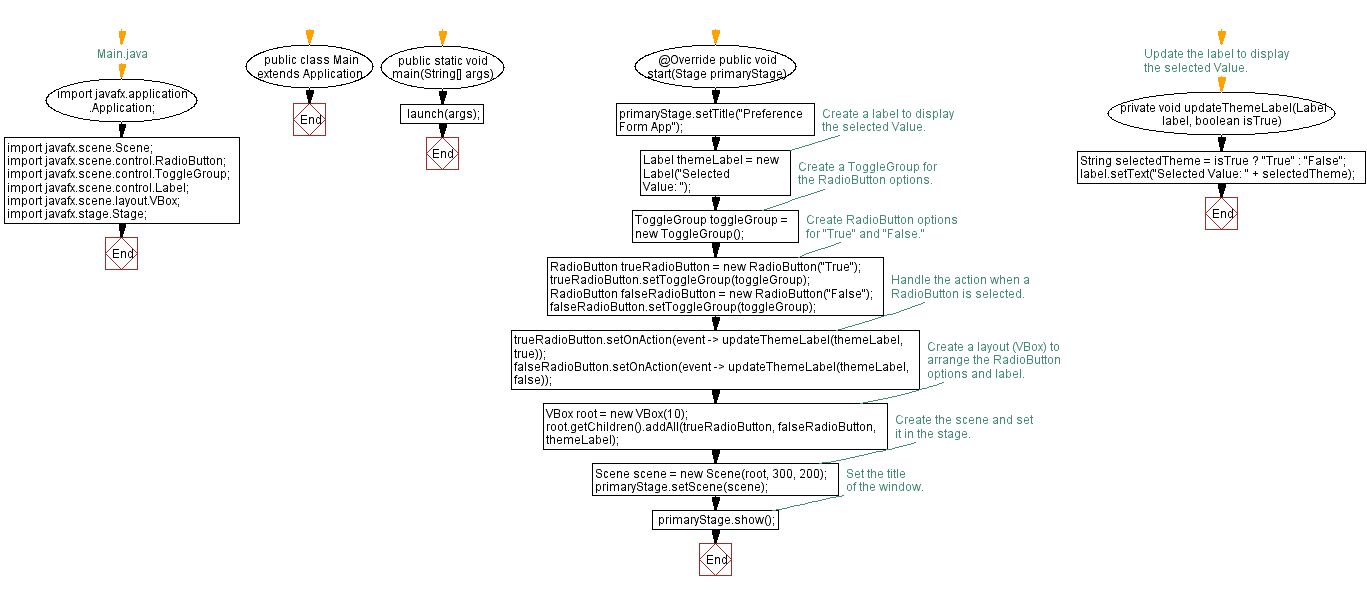
Go to:
PREV : CheckBox Selected Colors Display.
NEXT : Food Order with CheckBox and Cost.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.