JavaFX BorderPane different alignments
7. BorderPane with Custom Element Alignments
Write a JavaFX application that uses a BorderPane, and set different alignments for the top, center, and bottom elements.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("BorderPane with Different Alignments");
// Create a BorderPane layout
BorderPane borderPane = new BorderPane();
// Create labels for top, center, and bottom regions
Label topLabel = new Label("Top");
Label centerLabel = new Label("Center");
Label bottomLabel = new Label("Bottom");
// Set different alignments for the regions
BorderPane.setAlignment(topLabel, javafx.geometry.Pos.CENTER);
BorderPane.setAlignment(centerLabel, javafx.geometry.Pos.CENTER_LEFT);
BorderPane.setAlignment(bottomLabel, javafx.geometry.Pos.CENTER_RIGHT);
// Add labels to the BorderPane
borderPane.setTop(topLabel);
borderPane.setCenter(centerLabel);
borderPane.setBottom(bottomLabel);
// Create the scene and set it in the stage
Scene scene = new Scene(borderPane, 300, 200); // Width and height of the window
primaryStage.setScene(scene);
// Show the window
primaryStage.show();
}
}
In the exercise above, we create a 'BorderPane' layout and set different alignments for the top, center, and bottom elements using BorderPane.setAlignment. The 'topLabel' is aligned to the center, the 'centerLabel' is aligned to the center-left, and the 'bottomLabel' is aligned to the center-right. You can run this application to see the 'BorderPane' with different alignments.
Sample Output:
Flowchart:
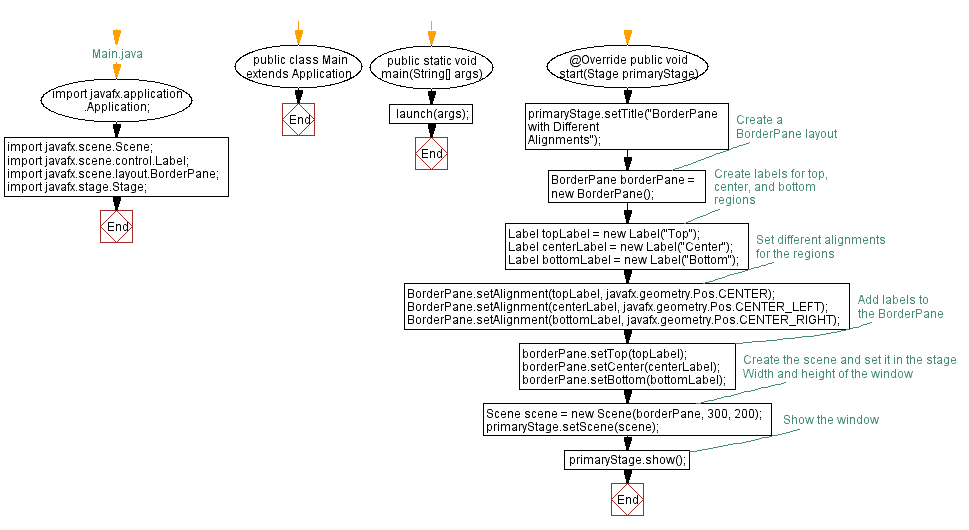
Go to:
PREV : Center-Aligned HBox Children.
NEXT : GridPane with Cell-Specific Alignment.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.