Horizontal Images: JavaFX application example
2. HBox with Horizontal Image Row
Write a JavaFX program that employs an HBox to place a set of images in a horizontal row.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Horizontal Images");
// Create Image objects for your images (provide the correct image file paths)
Image image1 = new Image("cpp-exercises.png");
Image image2 = new Image("java-exercises.png");
Image image3 = new Image("javafx-exercises.png");
// Create ImageView objects to display the images
ImageView imageView1 = new ImageView(image1);
ImageView imageView2 = new ImageView(image2);
ImageView imageView3 = new ImageView(image3);
// Create an HBox layout to arrange the images horizontally
HBox root = new HBox(10); // 10 is the spacing between images
root.getChildren().addAll(imageView1, imageView2, imageView3);
// Create the scene and set it in the stage
Scene scene = new Scene(root, 400, 200); // Width and height of the window
primaryStage.setScene(scene);
// Show the window
primaryStage.show();
}
}
In the above code, three images ('image1', 'image2', and 'image3') are loaded and displayed horizontally in an 'HBox' layout with a specified spacing between them. Run this application to see the images arranged in a horizontal row.
Sample Output:
Flowchart:
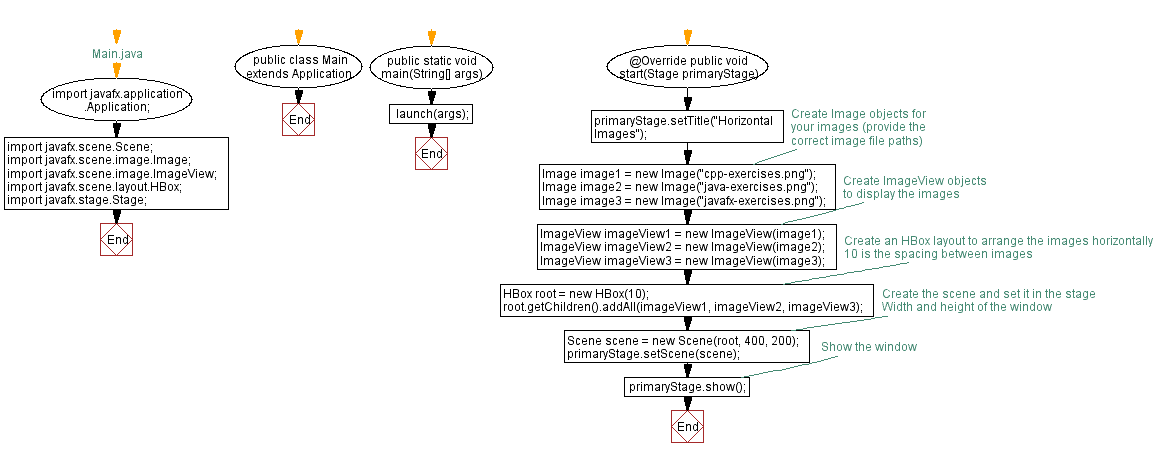
Go to:
PREV : VBox with Three Vertical Buttons.
NEXT : BorderPane with Label, Button, and ListView.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.