JavaFX keyboard input example
Write a JavaFX application that responds to keyboard input and displays the key pressed.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Key Press Example");
Label label = new Label("Press a key");
StackPane root = new StackPane(label);
Scene scene = new Scene(root, 300, 200);
scene.setOnKeyPressed(event -> {
String keyText = event.getText();
label.setText("Key pressed: " + keyText);
});
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the exercise above, the code creates a simple JavaFX application with a label that initially displays "Press a key." When you press any key on the keyboard, the label updates to show "Key pressed: [key]". Run this code to see how it responds to keyboard input.
Sample Output:
Flowchart:
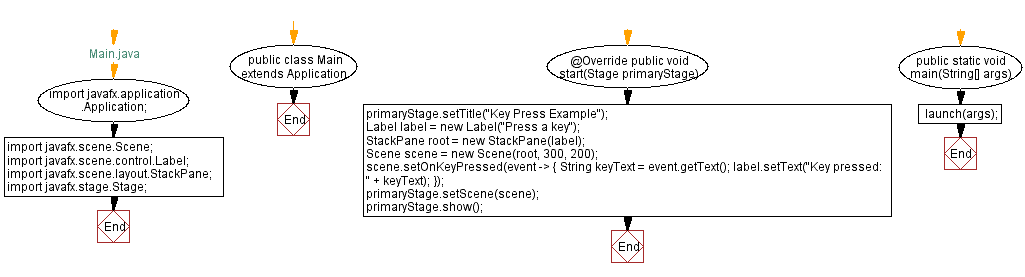
Java Code Editor:
Previous: JavaFX Mouse click application.
Next: JavaFX Key combination color change.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.