JavaFX Mouse click application
Write a JavaFX program that listens for mouse clicks and displays a message when the left mouse button is clicked.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import javafx.scene.layout.StackPane;
import javafx.scene.text.Text;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Mouse Click App");
Label clickLabel = new Label("Mouse Click: ");
// Create a VBox to arrange the labels
VBox vbox = new VBox(clickLabel);
// Create a scene
Scene scene = new Scene(vbox, 400, 300);
// Register a mouse clicked event handler
scene.setOnMouseClicked(event -> {
if (event.isPrimaryButtonDown()) {
//System.out.println("Hello\nAlexandra Abramov!");
clickLabel.setText("Mouse Click: Left button clicked");
}
});
primaryStage.setScene(scene);
primaryStage.show();
}
}
In the exercise above, we create a JavaFX application with a 'VBox' layout containing a 'Label' component to display the mouse click status. We then create a scene and register a mouse click event handler. The event handler checks if the left mouse button (primary button) is clicked and updates the 'clickLabel' accordingly.
Finally when we run the program and left-click the mouse, we will see the message "Mouse Click: Left button clicked."
Sample Output:
Flowchart:
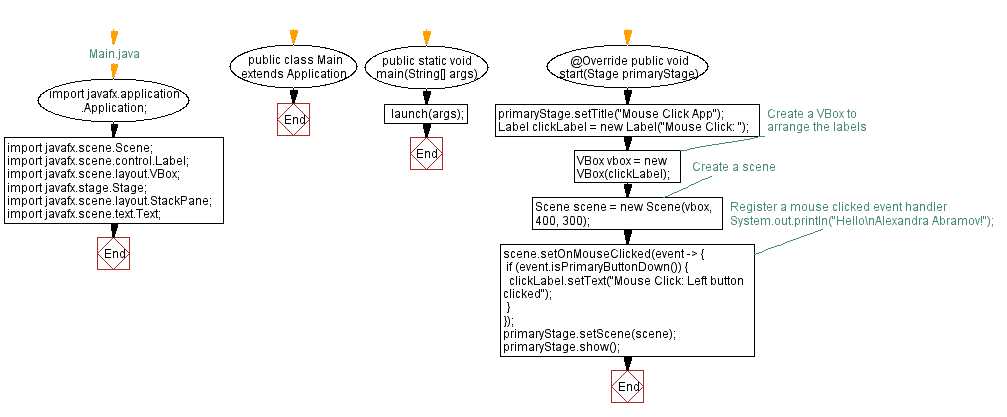
Java Code Editor:
Previous: JavaFX Mouse coordinates application.
Next: JavaFX keyboard input example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics