JavaFX event propagation game
Write a JavaFX program that builds a game where events propagate through various elements. Use event filters to handle game events efficiently.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
// Launch the JavaFX application
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Set the title for the game window
primaryStage.setTitle("Event Propagation Game");
// Create a root node
StackPane root = new StackPane();
// Create a button for the game
Button button = new Button("Click Me!");
// Add an event filter to the button to handle action events (button clicks)
button.addEventFilter(ActionEvent.ACTION, event -> {
System.out.println("Game Over: You clicked the button!");
event.consume(); // Prevent further event propagation
});
// Add the button to the root node
root.getChildren().add(button);
// Create the scene and set it on the stage
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
// Show the game window
primaryStage.show();
}
}
The above exercise demonstrates the concept of event propagation. In this simplified exercise, we create a game where you need to avoid clicking the button. When the button is clicked, the game ends and displays a "Game Over" message.
Sample Output:
Game Over: You clicked the button!
Flowchart:
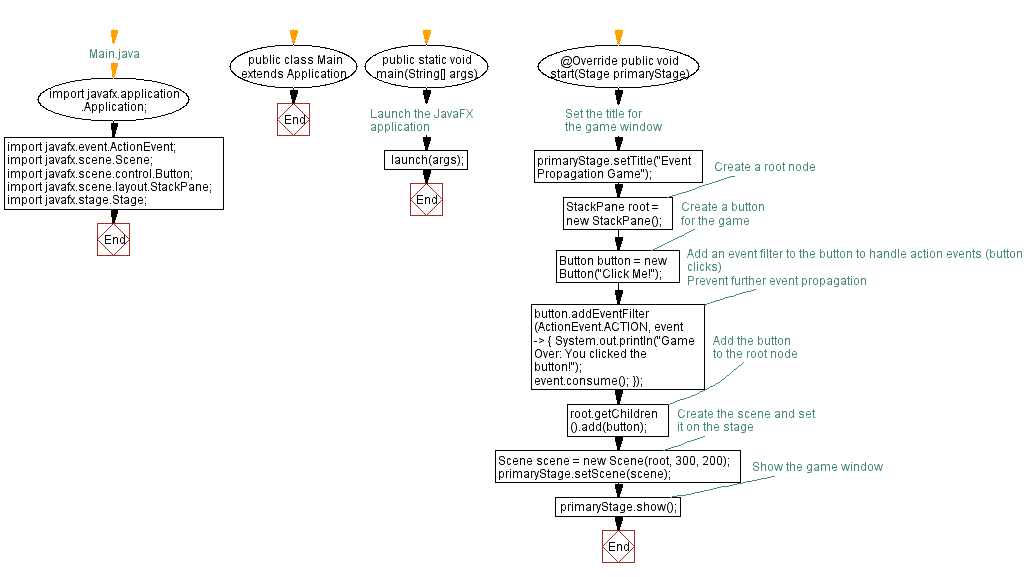
Java Code Editor:
Previous: JavaFX Tree-Like UI with event propagation.
Next: JavaFX drawing application with event filters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics