JavaFX Mouse coordinates application
JavaFx Events and Event Handling: Exercise-1 with Solution
Write a JavaFX program application that detects and displays mouse coordinates when moved over the application window.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Mouse Coordinates Application");
Label coordinatesLabel = new Label(" Mouse Coordinates: ");
Label currentCoordinatesLabel = new Label();
// Create a VBox to arrange the labels
VBox vbox = new VBox(coordinatesLabel, currentCoordinatesLabel);
// Create a scene
Scene scene = new Scene(vbox, 400, 300);
// Register a mouse moved event handler
scene.setOnMouseMoved(event -> {
double x = event.getSceneX();
double y = event.getSceneY();
currentCoordinatesLabel.setText(" X: " + x + ", Y: " + y);
});
primaryStage.setScene(scene);
primaryStage.show();
}
}
In the exercise above, we create a simple JavaFX application with a 'VBox' layout containing two 'Label' components. The first label, "Mouse Coordinates," is a static label, and the second label, "currentCoordinatesLabel," is used to display the mouse coordinates.
Next we create a scene and register a mouse move event handler for that scene. When the mouse is moved over the window, the event handler updates the 'currentCoordinatesLabel' with the current mouse coordinates.
Sample Output:
Flowchart:
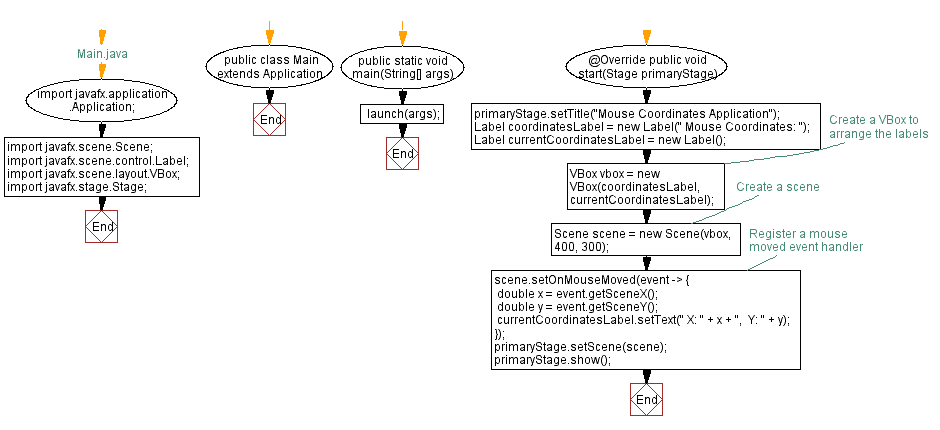
Java Code Editor:
Previous: JavaFx Events and Event Handling Exercises Home.
Next: JavaFX Mouse click application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/java-exercises/javafx/javafx-events-and-event-handling-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics