Event Handling: Creating a Clickable Circle in JavaFX
Write a JavaFX application with a circle that changes its color when clicked.
Sample Solution:
JavaFx Code:
Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Ellipse;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Color Changing Circle");
Pane root = new Pane();
// Create an Ellipse (circle).
Ellipse circle = new Ellipse(150, 150, 100, 100);
circle.setFill(Color.GREEN); // Initial color
// Handle the click event to change the circle's color.
circle.setOnMouseClicked(event -> {
if (circle.getFill() == Color.GREEN) {
circle.setFill(Color.RED);
} else {
circle.setFill(Color.GREEN);
}
});
root.getChildren().add(circle);
Scene scene = new Scene(root, 300, 300);
primaryStage.setScene(scene);
primaryStage.show();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Set the title of the application window to "Color Changing Circle."
- Create a 'Pane' named 'root' to serve as the root container for the application's UI elements.
- Create an 'Ellipse' named 'circle' with specific dimensions (center at [150, 150], major and minor radii of 100) to create a circle. The circle's initial color is set to green using circle.setFill(Color.GREEN).
- Handle the click event on the circle by using circle.setOnMouseClicked(...). The click event toggles the circle's color between green and red based on its current color.
- Add the circle to the 'root' container using root.getChildren().add(circle).
- Create a JavaFX Scene named scene with the root layout, setting its dimensions to 300x300 pixels.
- Set the created scene in the primary stage (primaryStage) and show the window.
Sample Output:
Flowchart:
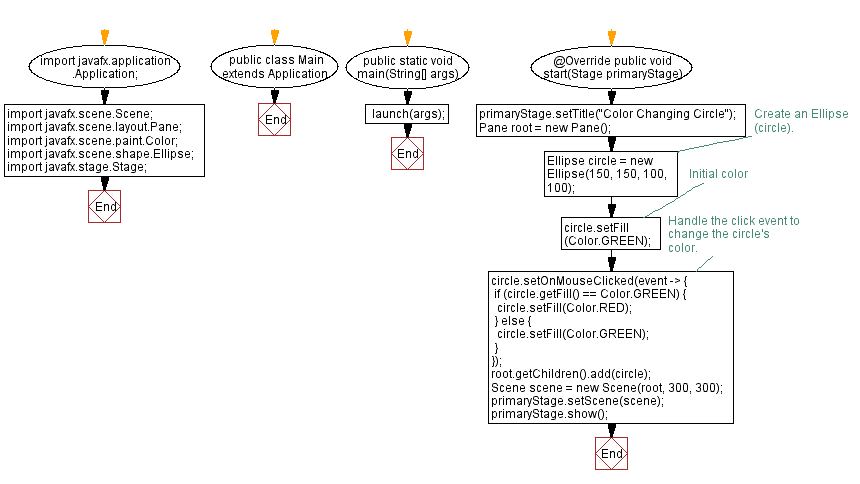
Java Code Editor:
Previous:Styling with CSS in JavaFX.
Next: Basic Animation: Creating a shape animation in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.