ChoiceBox and labels in JavaFX
5. ChoiceBox Changes Label Text
Write a JavaFX application that creates a ChoiceBox with a list of colors. Display a label that changes its text based on the selected color from the ChoiceBox.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.scene.Scene;
import javafx.scene.control.ChoiceBox;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a label to display the selected color.
Label colorLabel = new Label("Selected Color: ");
// Create a ChoiceBox with a list of colors.
ChoiceBox colorChoiceBox = new ChoiceBox<>(
FXCollections.observableArrayList(
"Red", "Green", "Blue", "Yellow", "Orange", "Purple"
)
);
// Set an action for the ChoiceBox.
colorChoiceBox.setOnAction(event -> {
String selectedColor = colorChoiceBox.getValue();
colorLabel.setText("Selected Color: " + selectedColor);
});
// Create a layout for the ChoiceBox and label.
VBox root = new VBox(10); // 10 is the spacing between elements.
root.getChildren().addAll(colorChoiceBox, colorLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200); // Width and height of the window.
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("Color Choice App");
// Show the window.
primaryStage.show();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Create a label with the initial text "Selected Color:."
- Create a ChoiceBox that contains a list of colors (Red, Green, Blue, Yellow, Orange, Purple). The "FXCollections.observableArrayList()" method is used to populate the ChoiceBox.
- Set an action for the ChoiceBox:
- When a color is selected, it updates the label's text to show the selected color.
- Organize the ChoiceBox and label in a vertical layout (VBox) with 10 pixels of spacing between them.
- Create a Scene with the layout, setting the window dimensions to 300x200.
- Set the window's title to "Color Choice App."
- Show the window.
Sample Output:
Flowchart:
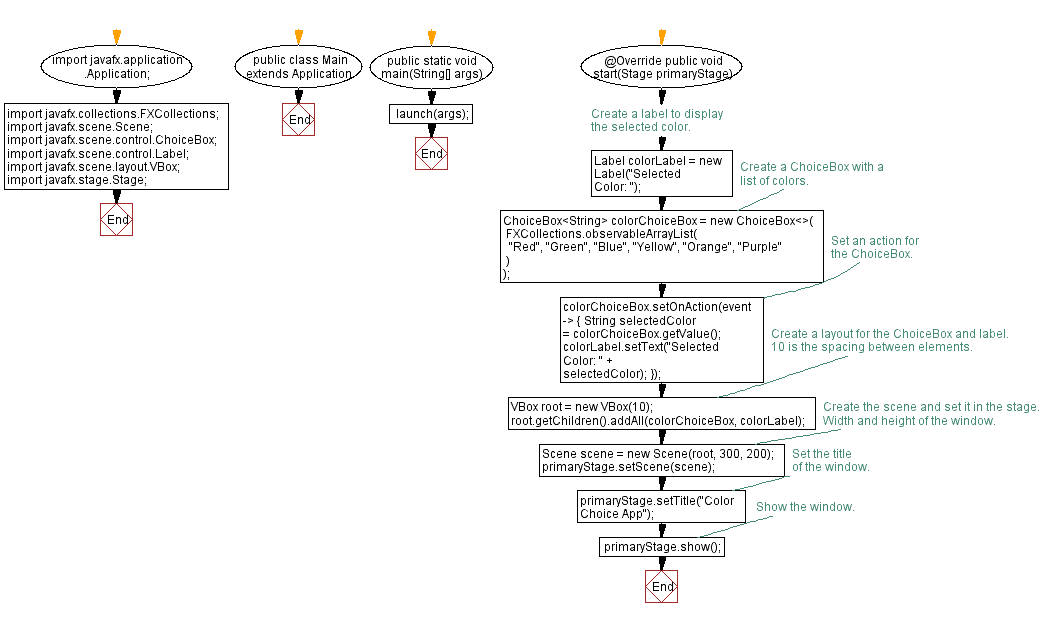
Go to:
PREV :Input Text to Label on Button Click.
NEXT : Load and Display Image.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.