Basic Animation: Creating a shape animation in JavaFX
10. Animate Shape on Button Click
Write a JavaFX application that animates a shape (e.g., a circle) to move across the window in response to a button click.
Sample Solution:
JavaFx Code:
Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
import javafx.animation.TranslateTransition;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Shape Animation");
Pane root = new Pane();
// Create a circle to animate.
Circle circle = new Circle(50, Color.RED);
circle.setCenterX(50);
circle.setCenterY(100);
// Create a button to start the animation.
Button startButton = new Button("Start Animation");
startButton.setLayoutX(150);
startButton.setLayoutY(250);
// Create a TranslateTransition for the circle.
TranslateTransition translateTransition = new TranslateTransition(Duration.seconds(2), circle);
// Set the target location for the animation.
translateTransition.setToX(250);
translateTransition.setAutoReverse(true);
translateTransition.setCycleCount(TranslateTransition.INDEFINITE);
// Handle the button click to start the animation.
startButton.setOnAction(event -> {
if (!translateTransition.getStatus().equals(TranslateTransition.Status.RUNNING)) {
translateTransition.play();
}
});
root.getChildren().addAll(circle, startButton);
Scene scene = new Scene(root, 300, 300);
primaryStage.setScene(scene);
primaryStage.show();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Set the title of the application window to "Shape Animation."
- Create a 'Pane' named 'root' to serve as the root container for the application's UI elements.
- Create a blue circle with a radius of 50, and set its initial position.
- Create a button labeled "Start Animation" and position it.
- Create a TranslateTransition named translateTransition to control the animation of the circle. The animation will move the circle from its initial position to the target position (toX).
- Handle the button click to start the animation. The animation is played only if it's not already running.
- Add the circle and the button to the root container.
- Create a JavaFX Scene named 'scene' with the 'root' layout, setting its dimensions to 300x300 pixels.
- Set the created scene in the primary stage (primaryStage) and show the window.
Sample Output:
Flowchart:
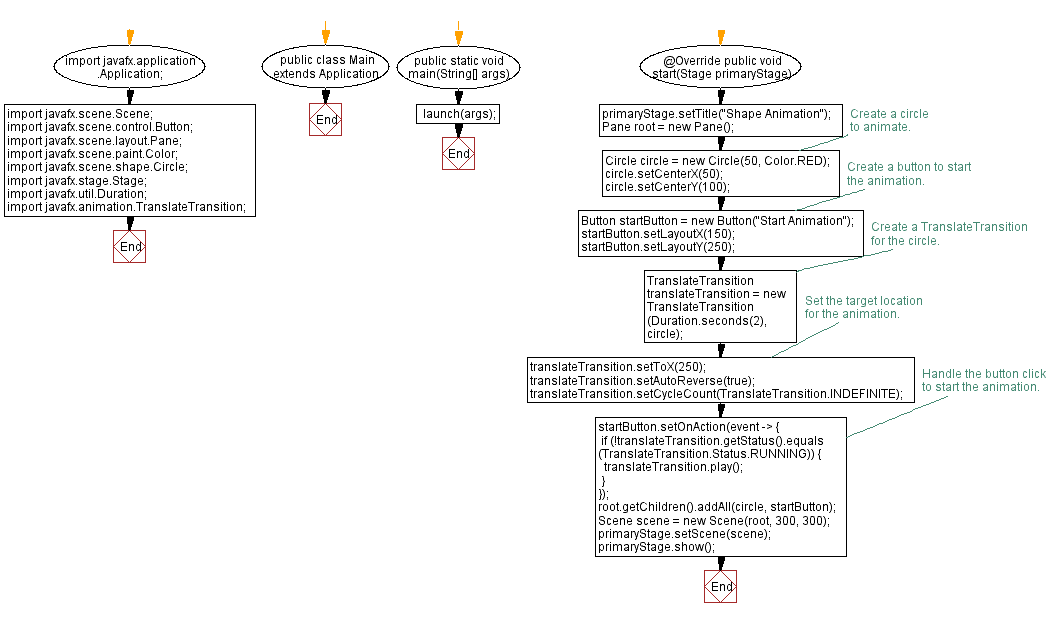
Go to:
PREV :Clickable Color Changing Circle.
NEXT : JavaFX UI Components Exercises Home.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.