Hello World JavaFX application
1. Display Hello JavaFX with Version
Write a JavaFX application that displays a "Hello, JavaFX!" message in a window. Your system's Java and JavaFX versions are displayed as well.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a label to display the "Hello, JavaFX!" message.
Label helloLabel = new Label(" Hello, JavaFX!");
// Create labels to display the Java and JavaFX versions.
Label javaVersionLabel = new Label(" Java Version: " + System.getProperty("java.version"));
Label javafxVersionLabel = new Label(" JavaFX Version: " + System.getProperty("javafx.version"));
// Create a VBox layout to arrange the labels vertically.
VBox root = new VBox(10); // 10 is the spacing between elements.
root.getChildren().addAll(helloLabel, javaVersionLabel, javafxVersionLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 150); // Width and height of the window.
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("Hello JavaFX App");
// Show the window.
primaryStage.show();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Create labels for "Hello, JavaFX!", Java version, and JavaFX version.
- Organize these labels in a vertical layout (VBox) with 10 pixels of spacing between them.
- Create a Scene with the layout, setting the window dimensions to 300x150.
- Set the window's title to "Hello JavaFX App."
- Display the window.
Sample Output:
Flowchart:
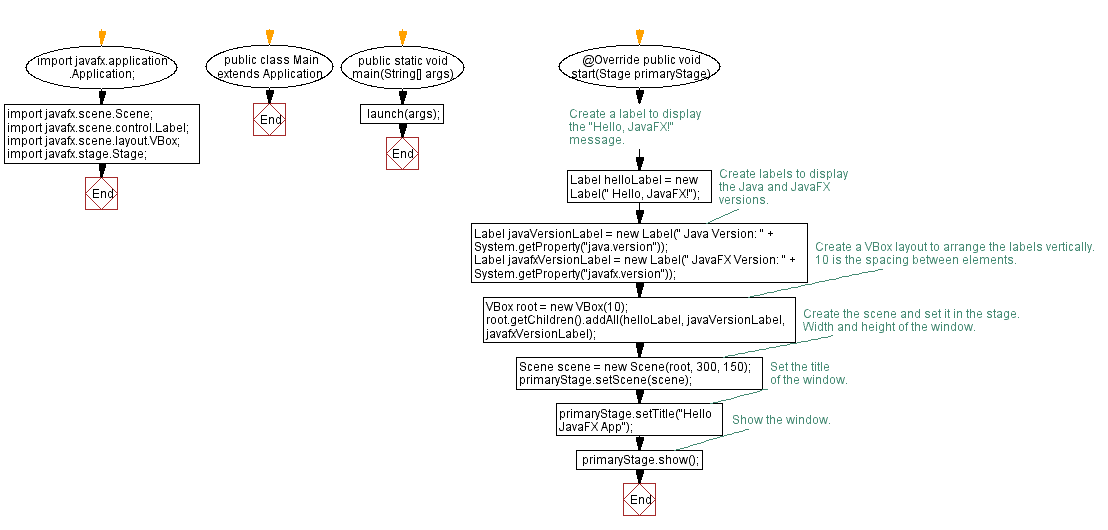
Go to:
PREV : JavaFx Basic Exercises Home.
NEXT : Button Click Shows Alert.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.