Java ProPolymorphism: Shape base class with Circle, Square, and Triangle subclasses
Java Polymorphism: Exercise-8 with Solution
Write a Java program to create a base class Shape with methods draw() and calculateArea(). Create three subclasses: Circle, Square, and Triangle. Override the draw() method in each subclass to draw the respective shape, and override the calculateArea() method to calculate and return the area of each shape.
Sample Solution:
Java Code:
//Shape.java
abstract class Shape {
public abstract void draw();
public abstract double calculateArea();
}
//Circle.java
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public void draw() {
System.out.println("Drawing a circle");
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
//Square.java
class Square extends Shape {
private double side;
public Square(double side) {
this.side = side;
}
@Override
public void draw() {
System.out.println("Drawing a square");
}
@Override
public double calculateArea() {
return side * side;
}
}
//Triangle.java
class Triangle extends Shape {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
@Override
public void draw() {
System.out.println("Drawing a triangle");
}
@Override
public double calculateArea() {
return 0.5 * base * height;
}
}
//Main.java
public class Main {
public static void main(String[] args) {
Shape circle = new Circle(7.0);
Shape square = new Square(12.0);
Shape triangle = new Triangle(5.0, 3.0);
drawShapeAndCalculateArea(circle);
drawShapeAndCalculateArea(square);
drawShapeAndCalculateArea(triangle);
}
public static void drawShapeAndCalculateArea(Shape shape) {
shape.draw();
double area = shape.calculateArea();
System.out.println("Area: " + area);
}
}
Sample Output:
Drawing a circle Area: 153.93804002589985 Drawing a square Area: 144.0 Drawing a triangle Area: 7.5
Explanation:
In the above exercise -
- "Shape" is the base abstract class, with Circle, Square, and Triangle as its subclasses. Each subclass overrides the draw() method to provide their specific shape drawing implementation. It also overrides the calculateArea() method to calculate and return the area of each shape.
- In the "Main()" class, we have a static method drawShapeAndCalculateArea(Shape shape) that takes an object of the base class Shape as a parameter. Inside this method, we call the draw() and calculateArea() methods on the shape object. Since the drawShapeAndCalculateArea method takes a Shape type parameter, it can accept objects of all three subclasses: Circle, Square, and Triangle, thanks to polymorphism.
Flowchart:
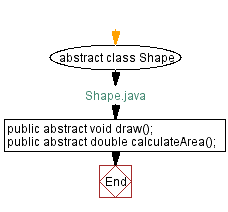
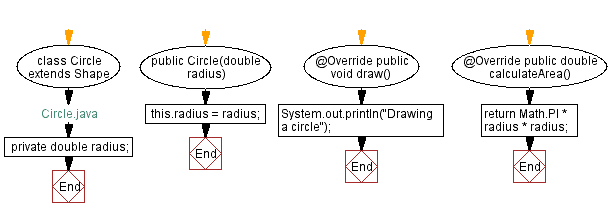
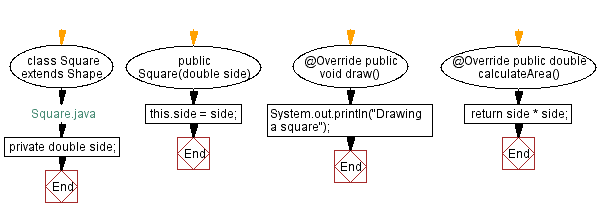
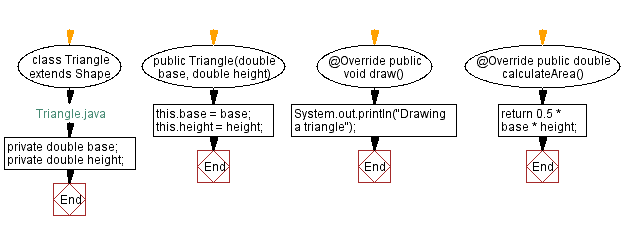
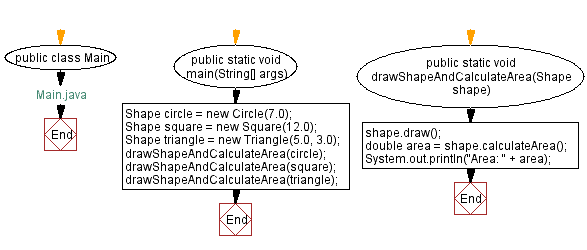
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Animal Base Class with Bird and Panthera Subclasses.
Next: BankAccount base class with Savings, Checking Account subclasses.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics