Java Polymorphism Programming - Vehicle Class with Car and Bicycle Subclasses for Speed Control
Java Polymorphism: Exercise-2 with Solution
Write a Java program to create a class Vehicle with a method called speedUp(). Create two subclasses Car and Bicycle. Override the speedUp() method in each subclass to increase the vehicle's speed differently.
In the given exercise, here is a simple diagram illustrating polymorphism implementation:
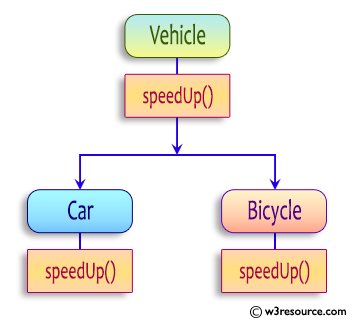
In the above diagram, Vehicle is the base class, and Car and Motorcycle are the subclasses. The arrow represents the inheritance relationship, indicating that Car and Motorcycle inherit from Vehicle.
Since both Car and Motorcycle are subclasses of Vehicle, they inherit the speed field and the speedUp() method. However, they override the speedUp() method to provide their own implementation.
This polymorphic relationship allows objects of type Car and Motorcycle to be treated as objects of type Vehicle. This means that you can use a Car or Motorcycle object where a Vehicle object is expected, which enables flexibility and code reuse.
Sample Solution:
Java Code:
// Vehicle.java
// Base class Vehicle
class Vehicle {
private int speed;
public void speedUp() {
speed += 10;
}
public int getSpeed() {
return speed;
}
}
// Car.java
// Subclass Car
class Car extends Vehicle {
@Override
public void speedUp() {
super.speedUp();
System.out.println("\nCar speed increased by 22 units.");
}
}
// Motorcycle.java
// Subclass Motorcycle
class Motorcycle extends Vehicle {
@Override
public void speedUp() {
super.speedUp();
System.out.println("Motorcycle speed increased by 12 units");
}
}
// Main.java
// Main class
public class Main {
public static void main(String[] args) {
Car car = new Car();
Motorcycle motorcycle = new Motorcycle();
System.out.println("Car initial speed: " + car.getSpeed());
System.out.println("Motorcycle initial speed: " + motorcycle.getSpeed());
car.speedUp();
motorcycle.speedUp();
System.out.println("\nCar speed after speeding up: " + car.getSpeed());
System.out.println("Motorcycle speed after speeding up: " + motorcycle.getSpeed());
}
}
Sample Output:
Car initial speed: 0 Motorcycle initial speed: 0 Car speed increased by 22 units. Motorcycle speed increased by 12 units Car speed after speeding up: 10 Motorcycle speed after speeding up: 10
Flowchart:
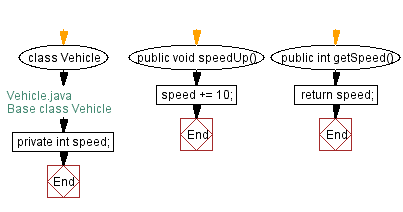
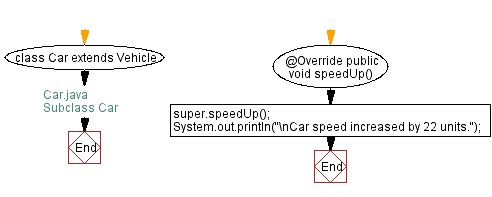
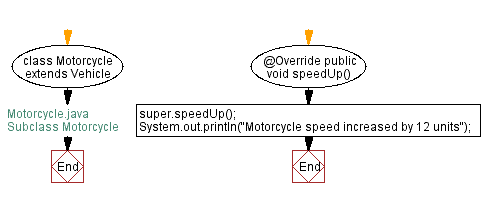
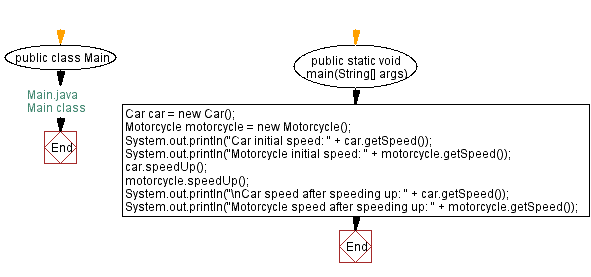
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Animal Class with Bird and Cat Subclasses for Specific Sounds.
Next: Shape Class with Circle, Rectangle, and Triangle Subclasses for Area Calculation.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics