Java Polymorphism: Animal base class with Lion, Tiger, and Panther subclasses
Write a Java program to create a base class Animal with methods eat() and sound(). Create three subclasses: Lion, Tiger, and Panther. Override the eat() method in each subclass to describe what each animal eats. In addition, override the sound() method to make a specific sound for each animal.
Sample Solution:
Java Code:
Output:
Lion eats meat. Lion roars. Tiger eats meat and sometimes fish. Tiger growls. Panther eats meat and small mammals. Panther purrs and sometimes hisses.
Explanation:
In the above exercise -
- The "Animal" class is the base class and 'Lion', 'Tiger', and 'Panther' are its subclasses. Each subclass overrides the eat() method to describe what each animal eats and the sound() method to make a specific sound for each animal.
- In the main() method, we create instances of Lion, Tiger, and Panther. We then call the eat() and sound() methods on these objects. Since these methods are overridden in the subclasses, the appropriate behavior for each animal will be displayed when we run the program.
Flowchart:
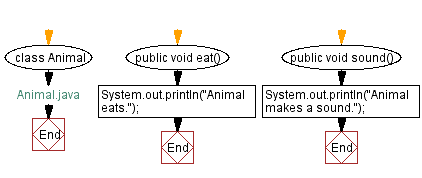
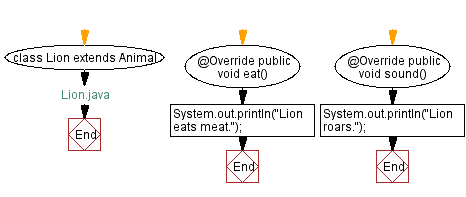
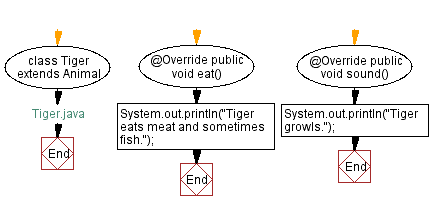
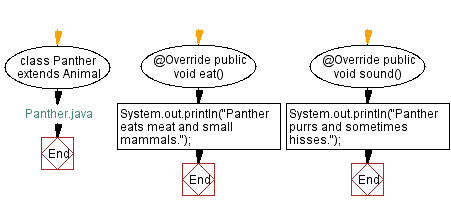
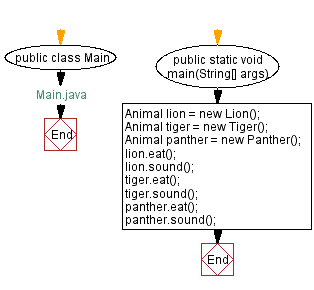
For more Practice: Solve these Related Problems:
- Write a Java program where the "Animal" class categorizes animals based on their diet (herbivore, carnivore, omnivore).
- Write a Java program where the "Animal" class allows animals to communicate with each other based on their sounds.
- Write a Java program where the "Animal" class tracks daily food consumption.
- Write a Java program where the "Animal" class supports hibernation behavior.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: BankAccount base class with Savings, Checking Account subclasses.
Next: Vehicle base class with Car and Motorcycle subclasses.
What is the difficulty level of this exercise?