Java Interface: Implement the Flyable interface
Java Interface: Exercise-3 with Solution
Write a Java program to create an interface Flyable with a method called fly_obj(). Create three classes Spacecraft, Airplane, and Helicopter that implement the Flyable interface. Implement the fly_obj() method for each of the three classes.
Sample Solution:
Java Code:
// Flyable.java
// Interface Flyable
// Declare the Flyable interface
interface Flyable {
// Declare the abstract method "fly_obj" that classes implementing this interface must provide
void fly_obj();
}
// Spacecraft.java
// Class Spacecraft
// Declare the Spacecraft class, which implements the Flyable interface
class Spacecraft implements Flyable {
// Implement the "fly_obj" method required by the Flyable interface
@Override
public void fly_obj() {
// Print a message indicating that the Spacecraft is flying
System.out.println("Spacecraft is flying");
}
}
// Airplane.java
// Class Airplane
// Declare the Airplane class, which implements the Flyable interface
class Airplane implements Flyable {
// Implement the "fly_obj" method required by the Flyable interface
@Override
public void fly_obj() {
// Print a message indicating that the Airplane is flying
System.out.println("Airplane is flying");
}
}
// Helicopter.java
// Class Helicopter
// Declare the Helicopter class, which implements the Flyable interface
class Helicopter implements Flyable {
// Implement the "fly_obj" method required by the Flyable interface
@Override
public void fly_obj() {
// Print a message indicating that the Helicopter is flying
System.out.println("Helicopter is flying");
}
}
// Main.java
// Class Main
// Declare the Main class
public class Main {
public static void main(String[] args) {
// Create an array of Flyable objects, including a Spacecraft, Airplane, and Helicopter
Flyable[] flyingObjects = {new Spacecraft(), new Airplane(), new Helicopter()};
// Iterate through the array and call the "fly_obj" method on each object
for (Flyable obj : flyingObjects) {
obj.fly_obj();
}
}
}
Sample Output:
Spacecraft is flying Airplane is flying Helicopter is flying
Flowchart of Interface Flyable:
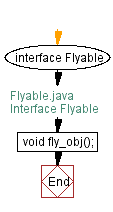
Flowchart of Class Spacecraft:
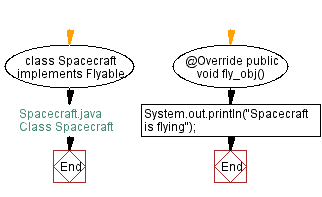
Flowchart of Class Airplane:
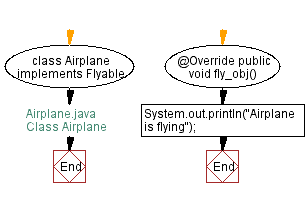
Flowchart of Class Helicopter:
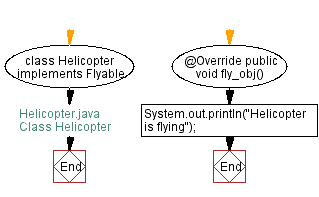
Flowchart of Class Main:
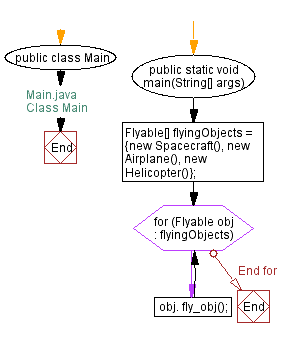
Java Code Editor:
Previous: Shape with the getArea() method, implement the Shape interface.
Next: Banking system classes - Bank Account, Savings Account, and Current Account.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics