Java Inheritance Programming - Animal with a method called makeSound
Write a Java program to create a class called Animal with a method called makeSound(). Create a subclass called Cat that overrides the makeSound() method to bark.
This exercise shows how inheritance works in Java programming language. Inheritance allows you to create new classes based on existing classes, inheriting their attributes and behaviors. In this case, the 'Cat' class is a more specific implementation of the 'Animal' class, adding quarrel behavior.
Sample Solution:
Java Code:
// Define a public class named Animal
public class Animal {
// Define a public method named makeSound
public void makeSound() {
// Print "The animal makes a sound." to the console
System.out.println("The animal makes a sound.");
}
}
The above code defines a Java class named "Animal". Within this class, there is a method called "makeSound()". When this method is called, it prints the message "The animal makes a sound." to the console. Essentially, this class serves as a blueprint for creating objects representing generic animals that can make a sound. However, the specific sound is not defined here.
// Define a public class named Cat that extends Animal
public class Cat extends Animal {
// Use the @Override annotation to indicate that this method overrides a method in the superclass
@Override
// Define the makeSound method
public void makeSound() {
// Print "The cat quarrels." to the console
System.out.println("The cat quarrels.");
}
}
The above code defines a Java class named "Cat" that extends another class called "Animal". Within the "Cat" class, there is a method called "makeSound()" that overrides the same method from the "Animal" class. When this method is called for a 'Cat' object, it prints the message "The cat quarrels." to the console. This means that 'Cat' is a specific type of 'Animal' and has its own unique sound, which is defined in this method.
// Define a public class named Main
public class Main {
// Define the main method
public static void main(String[] args) {
// Create an instance of Animal
Animal animal = new Animal();
// Create an instance of Cat
Cat cat = new Cat();
// Call the makeSound method on the animal instance
animal.makeSound();
// Call the makeSound method on the cat instance
cat.makeSound();
}
}
The above code defines a Java class named 'Main' with a 'main' method. Inside the 'main' method:
An 'Animal' object named 'animal' is created.
A 'Cat' object named 'cat' is created.
Both 'Animal' and 'Cat' are classes defined elsewhere in the code. They have a method called 'makeSound()'.
Then, the 'makeSound()' method is called on both the 'animal' and 'cat' objects:
'animal.makeSound()' calls the 'makeSound()' method of the 'Animal' class, which prints "The animal makes a sound." to the console.
'cat.makeSound()' calls the overridden 'makeSound()' method of the 'Cat' class, which prints "The cat quarrels." to the console.
Output:
The animal makes a sound. The cat quarrels.
Explanation:
The above exercise demonstrates Java programming inheritance. In this program, we create a base class called 'Animal' with a method named makeSound(). Then, you will create a subclass of 'Animal' called 'Cat' which inherits from 'Animal'. The 'Cat' class will override the makeSound() method and change it to make a quarrel sound.
Flowchart:
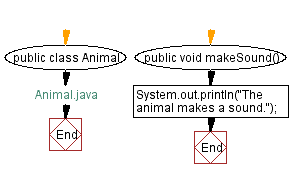
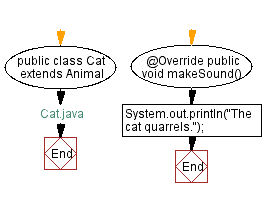
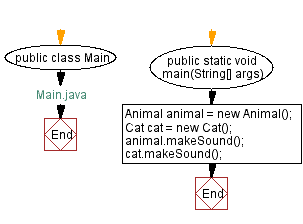
For more Practice: Solve these Related Problems:
- Write a Java program where the "Cat" subclass adds a new method called scratch() that prints a message when called.
- Write a Java program where the "Animal" class includes an attribute for habitat, and subclasses define specific habitats.
- Write a Java program where the "Cat" subclass overrides the makeSound() method to purr instead of meowing.
- Write a Java program where the "Animal" class has a lifespan attribute, and subclasses override it based on species.
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Java Inheritance Exercises Home.
Next: Create a class called Vehicle with a method called drive(). Create a subclass called Car that overrides the drive() method to print "Repairing a car".
What is the difficulty level of this exercise?